A Python translator is a program where we can receive text from the user as input and then the user can input the type of language in which he/she wants to translate the text.
This program can be created on behalf of Python only but that would be unnecessarily complex and time-consuming. This type of problem is faced in many programs. To save time and avoid complexity, we will use googletrans, a Python library with an inbuilt function to translate text. Python has more than 1 lakh libraries to perform different tasks.
1. Introduction to Python Translator:
In this program, We are going to create a Python program that will receive a text from the user and then ask for the language in which it needs to be translated. The user inputs the language at the end of the program and the program generates the translated text.
To create the program, we need to install the library i.e. googletrans

In your terminal type “pip install googletrans” and press enter.

If you face any issues in installation, try “pip install googletrans==3.1.0a0“
————–you are reading: 6 easy steps to create a Python translator————
2. Importing the translator function from the library
In your editor screen, let’s start coding.
from googletrans import Translator()
Here, googletrans is a library and it has a class called Translator() which we are importing to perform the task.
3. Creating a function
Let’s create a function that will translate the text and return the text to the new language.
def translate_text(text, target_language='en'):
translator = Translator()
translation = translator.translate(text, dest=target_language)
return translation.text
A. translate_text is a function defined by the user that receives the user’s text as an argument and the language of the text is ‘en’ for English.
B. translator is an object of the class Translator
C. In this line the text will be translated into the destination language(as the user wants)
D. Finally the function, returns the translated text
————–you are reading: 6 easy steps to create a Python translator————
4. Receiving Text & Language from User
text_to_translate = input("Enter the text to translate: ")
target_language = input("Enter the target language (e.g., 'hi' for hindi): ")
A. text_to_translate is a variable that takes text as input from the user.
B. target_language is a variable that takes the target language from the user(in which the user wants the text to be translated)
5. Calling the function
translated_text = translate_text(text_to_translate, target_language)
In the end, we call the function translate_text with text_to_translate and target_language as arguments and the returned output will be stored in translated_text(variable).
————–you are reading: 6 easy steps to create a Python translator————
6. Print the output
print(f"\nOriginal Text: {text_to_translate}")
print(f"Translated Text : {translated_text}")
Now is the time to print the outputs( original text and the translated text.)
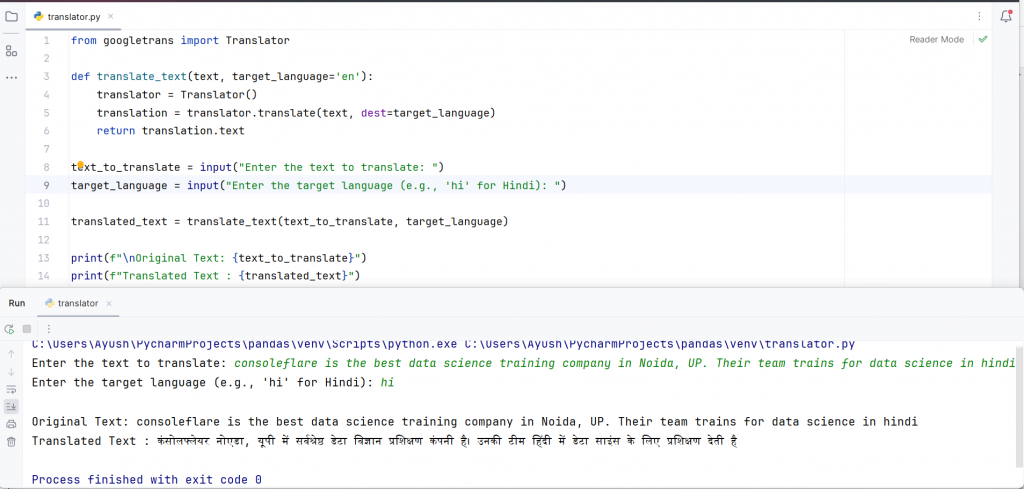
Want to know, What else we can do with Python?
Follow us on Facebook, LinkedIn, Instagram
Apart from Python translator, we all know that Python is a general-purpose programming language which means Python can be used in various fields like data science, web development, game development, IOT(Internet of Things), etc.
If you wish to learn more about data science or want to curve your career in the data science field feel free to join our free workshop on Masters in Data Science with PowerBI, where you will get to know how exactly data science fieldwork and why companies are ready to pay handsome salaries in this field.
In this workshop, you will get to know each tool and technology from scratch that will make you skillfully eligible for any data science profile.
To join this workshop, register yourself on consoleflare and we will call you back.