Python String Methods
So for all the coders, who just started Python, Python String methods can be overwhelming. There are so many Python string methods that it is very easy to lose count and get confused. This blog will cover all Python String methods in one So you need not google every time you are stuck.
Python String Methods Python String Methods Python String Methods Python String Methods Python String Methods Python String Methods
lower() Method:
lower() method is used to return a string with all its character in lowercase. We do not pass any parameters in lower().
company = "CONSOLE FLARE"
var = company.lower()
print(var)
Output : console flare
upper() Method:
upper() method is used to return a string with all its characters in the Capital case. We do not pass any parameters in upper().
company = "console flare"
var = company.upper()
print(var)
Output : CONSOLE FLARE
swapcase() Method:
swapcase() method is used to return string with all its characters in lower to upper case and all its string in upper to lower case. It does not have any parameters.
company = "CoNSole FLAre"
var = company.swapcase()
print(var)
Output : cOnsOLE flaRE
title() Method:
The title () method in python is used to convert the first character in each word to Uppercase and the remaining characters to Lowercase in the string and returns a new string. It does not have any parameters.
sentence = "pyThon is a geneRAL PuRPOSE programming Language."
var = sentence.title()
print(var)
Output : Python Is A General Purpose Programming Language.
capitalize() Method:
capitalize() method return string with its first character as upper case and rest in lower case. It does not have any parameters.
sentence = "pyThon is a geneRAL PuRPOSE programming Language.
var = sentence.capitalize()
print(var)
Output : Python is a general purpose programming language.
strip(),lstrip() and rstrip() Method:
strip() method removes all the whitespace from the left and right sides of the string.
company = " console flare "
var = company.strip()
print(company)
print(var)
Output : console flare
console flare
lstrip() method removes all the whitespace from the left side of the string.
company = " console flare "
var = company.lstrip()
print(company)
print(var)
Output : console flare
console flare
rstrip() method removes all the whitespace from the right side of the string.
company = "console flare "
var = company.rstrip()
print(company)
print(var)
Output : console flare
console flare
replace() Method:
replace() method returns a string where a specified value is replaced with a specified value.
Parameters to be passed in replace:
old value: The string to be replaced. It is Required
new value: The string to replace the old value with. It is Required
count: An integer value specifying how many occurrences of the old string you want to replace. The default value of the count is All Occurences. It is Optional.
replace(oldvalue,newvalue,count)
sentence = "I am learning C Language C Language."
To replace ‘C Language’ with ‘Python Programming Language’, we will use replace() method.
sentence = sentence.replace('C Language','Python Programming Language')
print(sentence)
Output : I am learning Python Programming Language Python Programming Language.
To see, How count works, I will be using the count parameter below example:
sentence = "I am learning C Language C Language."
sentence = sentence.replace('C Language','Python Programming Language',1)
print(sentence)
Output : I am learning Python Programming Language C Language.
count() method:
count() method returns the number of times a specified value occurs in a string.
Parameters to be passed in count():
value: substring or value to count. It is Required.
start index: The position from where to start the search. It is Optional. The default value is 0.
end index: The position from where to end the search. It is Optional. The default value is the end of the string.
count(value,start,end)
sentence = "Python is Python and C is C"
var = sentence.count("Python")
print(var)
Output : 2
To see, How start and end parameters work, i will be using them in below example:
sentence = "Python is Python and C is C"
var = sentence.count("Python",1,16)
print(var)
Output : 1
find() Method:
find() method searches the string for a specified value and returns the starting position of where it is found. It searches the whole string for specified values from left to right.
In simpler terms, it finds the string’s position.
Parameters to be passed inside find() method:
value: The values to search for. It is Required.
start: Starting index, from where to look for the value. It is Optional
end: Ending index, from where to stop the search for value. It is Optional
find(value,start,end)
sentence = "Python is general purpose programming language."
lookfor = sentence.find("Python")
print(lookfor)
Output : 0
To see, How start and end parameters work , i will be using them in below example:
sentence = "Python is Python and C is C"
lookfor = sentence.find("Python",1,19)
print(lookfor)
Output : 10
Hence, the operation of start and end is the same everywhere, it looked for ‘python’ from index number 1 to 18.
rfind() method:
rfind() method is the same as the find() method with the same parameters. The only difference is it looks for a specified string from right to left.
sentence = "Python is Python and C is C"
lookfor = sentence.rfind("Python")
print(lookfor)
Output : 10
index() and rindex() method:
index() method also searches the string for a specified value and returns the position of where it is found.
In more simpler terms index() works like find() and rindex() works like rfind(). They both have the same parameters.
sentence = "Python is Python and C is C"
lookfor = sentence.index("Python")
print(lookfor)
Output : 0
sentence = "Python is Python and C is C"
lookfor = sentence.rindex("Python")
print(lookfor)
Output : 10
Difference between find() and index() Method:
find() does not give an error when value or substring is not found and the flow of the program does not interrupt.
sentence = "Python is Python and C is C"
lookfor = sentence.find("Java")
print(lookfor)
Output : -1
index() gives an error when a value or substring is not found.
sentence = "Python is Python and C is C"
lookfor = sentence.index("Java")
print(lookfor)
Error : ValueError Traceback (most recent call last)
<ipython-input-55-7829979dbdb0> in <module>
1 sentence = "Python is Python and C is C"
----> 2 lookfor = sentence.index("Java")
3 print(lookfor)
ValueError: substring not found
split() and rsplit() Method:
split() method splits the string at the specified separator and returns a list.
Parameters to be passed in split():
separator: Specifies the separator to use when splitting the string. It is Optional. By Default, any whitespace is the separator.
maxsplit : maxsplit specifies how many splits to do. It is Optional.Its Default value is -1 or All Occurences
sentence = "Python is a general purpose programming language"
var = sentence.split(" ")
print(var)
Output : ['Python', 'is', 'a', 'general', 'purpose', 'programming', 'language']
To see, How maxsplit work, i will be using them in the below example:
sentence = "Python is a general purpose programming language"
var = sentence.split(" ",2)
print(var)
Output : ['Python', 'is', 'a general purpose programming language']
rsplit() works like split(), it also splits the string from right to left. For Example:
sentence = "Python is a general purpose programming language"
var = sentence.rsplit(" ")
print(var)
Output : ['Python', 'is', 'a', 'general', 'purpose', 'programming', 'language']
Difference between split() and rsplit():
if you use split() and rsplit() method on a same string you will find there is no difference in output. let’s use them both on a single string:
sentence = "This is Console Flare Python class"
var = sentence.split(" ")
print(var)
Output : ['This', 'is', 'Console', 'Flare', 'Python', 'class']
sentence = "This is Console Flare Python class"
var = sentence.rsplit(" ")
print(var)
Output : ['This', 'is', 'Console', 'Flare', 'Python', 'class']
split() splits the string from left to right and rsplit() splits the string from right to left, yet output is same always.
Difference between split() and rsplit() can only be shown with maxsplit(). let’s follow these examples:
sentence = "This is Console Flare Python class"
var = sentence.split(" ",2)
print(var)
Output : ['This', 'is', 'Console Flare Python class']
sentence = "This is Console Flare Python class"
var = sentence.rsplit(" ",2)
print(var)
Output : ['This is Console Flare','Python','class']
Because rsplit() splits the string from right to left, maxsplit gives a different result from split() method.
partition() and rpartition() method:
partition() method returns a string where the string is parted into three parts:
- 1.Everything before the first separator
- 2.Separator itself.
- 3.Everything after the first separator.
Parameter to be passed into method: separator
partition(separator)
sentence = "python is Cool"
var = sentence.partition(" ")
print(var)
Output : ('python', ' ', 'is Cool')
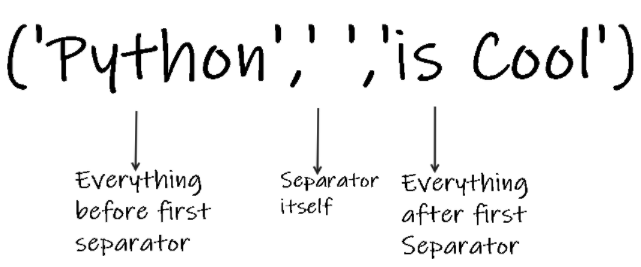
rpartition() is same as partition() , only it starts from right to left.
sentence = "python is Cool"
var = sentence.rpartition(" ")
print(var)
Output : ('python is', ' ', 'Cool')
Methods that returns only Boolean Values(True or False):
islower() method:
islower() returns True if all the characters in a string are lowercase, otherwise, it returns False. It has no Parameters.
company = 'console flare'
var = company.islower()
print(var)
Output : True
isupper() method:
isupper() returns True if all the characters in a string are uppercase, otherwise, it returns False. It has no Parameters.
company = 'CONSOLE FLARE'
var = company.isupper()
print(var)
Output : True
isalpha() method:
isalpha() returns True if all the characters in a string are only alphabets, otherwise, it returns False. It has no Parameters.
company = 'CONSOLEFLARE'
var = company.isalpha()
print(var)
Output : True
isnumeric() method:
isnumeric() returns True if all the characters in a string are only numbers, otherwise, it returns False. It has no Parameters.
num = "15"
var = num.isnumeric()
print(var)
Output : True
isalnum() method:
isalnum() returns True if all the characters in a string are either number or string or both, otherwise, it returns False. It has no Parameters.
num = "123abc"
var = num.isalnum()
print(var)
Output : True
startswith() method:
startswith() returns true if the string starts with a specified value.
Parameters to be Passed in method:
value: The values to check if the string starts with. It is Required
start: An integer specifying at which position to start the search. It is Optional
end: An integer specifying at which position to end the search. It is Optional
name = "Console flare"
var = name.startswith("Con")
print(var)
Output : True
endswith() method:
endswith() returns true if the string ends with a specified value.
Parameters to be Passed in method:
value: The values to check if the string ends with. It is Required
start: An integer specifying at which position to start the search. It is Optional
end: An integer specifying at which position to end the search. It is Optional
name = "Console flare"
var = name.endswith("e")
print(var)
Output : True
Python String Methods is one of the biggest tools When performing data science, we deal with textual data a lot. Moreover, the textual data requires much more preprocessing than plain numbers. Thankfully, Python string methods are capable of performing such tasks efficiently and smoothly.
I have tried to cover most of the important Python String Methods, and if I missed any, do tell and leave a comment below or for any query reach us at ConsoleFlare.
Hello there! This is kind of off topic but I need some help from an established blog. Is it hard to set up your own blog? I’m not very techincal but I can figure things out pretty fast. I’m thinking about making my own but I’m not sure where to start. Do you have any points or suggestions? Many thanks