Python is a powerful multi-purpose programming language used by multiple giant companies. It has simple and easy-to-use syntax making it the perfect language for someone trying to learn computer programming for the first time
Introduction to Python’s Guess the Word Game
In this Project, we will create a program to create a guess the word game which will choose a secret or magic word and ask the user to guess the word by providing the letters. These letters provided must automatically be placed in the position where they are in the word so that the user may understand and guess the magic word.
Your Program must provide 10 chances for the user to guess and win the game.
Step 1: Importing the necessary module from Python.
In this step, we will import the random module and create a list of words that will be used for the guessing game.
import random as rd
#List of words for guessing
words = ['SEVEN', 'SNAKE', 'APPLE', 'MANGO', 'TOMB', 'JERRY', 'HELLO', 'HAPPY', 'BREAK']
Step 2: Creating a function
In this step, we will create a function by the name of print_place with the argument.
def print_place(underscores):
for i in underscores:
print(i, end=' ')
print()
The purpose of this function is to update the word again and again when the user inputs the correct alphabet.

For more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
Step 3: Printing the game instruction
In this step of the Python code, we will print the instructions so that the user can understand the rules and then proceed with the game.
#Printing the game instruction
print('--------Guess the Word Game---------------')
print('You Have 10 chances to win the game')
print('You have to guess the secret word using letters')
print('The letters will be placed automatically on the place if correct')
print("Let's Start----->")
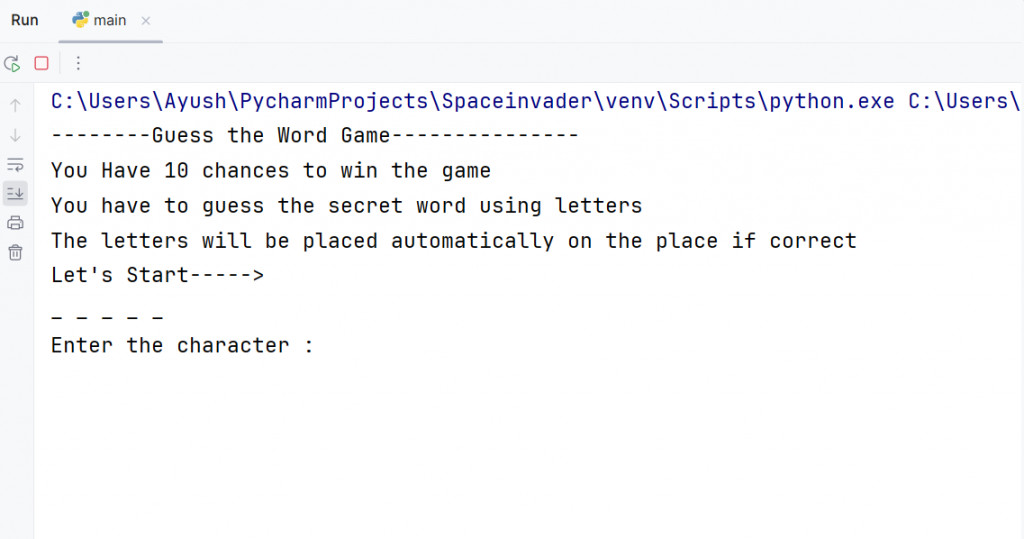
You are reading——-Python: Create Guess the Word game in 6 steps
Step 4: Select a word randomly from the list and create a list of underscores.
# selecting the word from the list of words
word = rd.choice(words)
#creation of a list for placing _ _ _ _ _
underscores = ['_' for i in range(len(word))]
#Printing the first time
print_place(underscores)
In this part of the Python code, we have used rd.choice(words) which will select a word from the words list randomly and store it in the variable word. Later on, we created a list of underscores like this (_ _ _ _ _ _ _ _ _) with the same name to give a dramatic essence and also an idea to the player so that the player can see how many more alphabets are left to guess. At last, we have called the print_place() function with the argument underscores and declared a variable win with the stored boolean value False stored in it.
We also recommend you read this blog on file handling for a better understanding of this project:
Nested Lists: 10 Easy Steps For Student Management System
Step 5: Create a loop to take alphabets one by one from the user and fix them.
#running the loop for executing the game with 10 chances.
for i in range(10):
char = input('Enter the character : ')
for i in range(len(word)):
if word.lower()[i] == char:
underscores[i] = char
print_place(underscores)
print()
if "".join(underscores) == word.lower():
print_place(underscores)
print('You Won the Game')
print('------------------------------------------')
break
else:
print('Try Again!!')
Now we create a loop that will run 10 times because this is the number of chances which a user gets to guess the alphabet. Inside this loop, we receive an input and store it in a variable named char. Now we run a loop to check if the alphabet entered by the user is equal to the alphabet stored in the i index number of the word. If it is true then this alphabet will be stored in the i index number of the underscores list.
Then we will call the print_place() function with the underscore as an argument which will print the list with underscores and the alphabet stored recently so that the user can see how many more alphabets are yet to guess. After that, we used conditional statements to whether the word selected by the computer matches the word inputted by the user if the condition is true, it will display the message “You won the game” and will break the loop otherwise it will show “Try Again”.
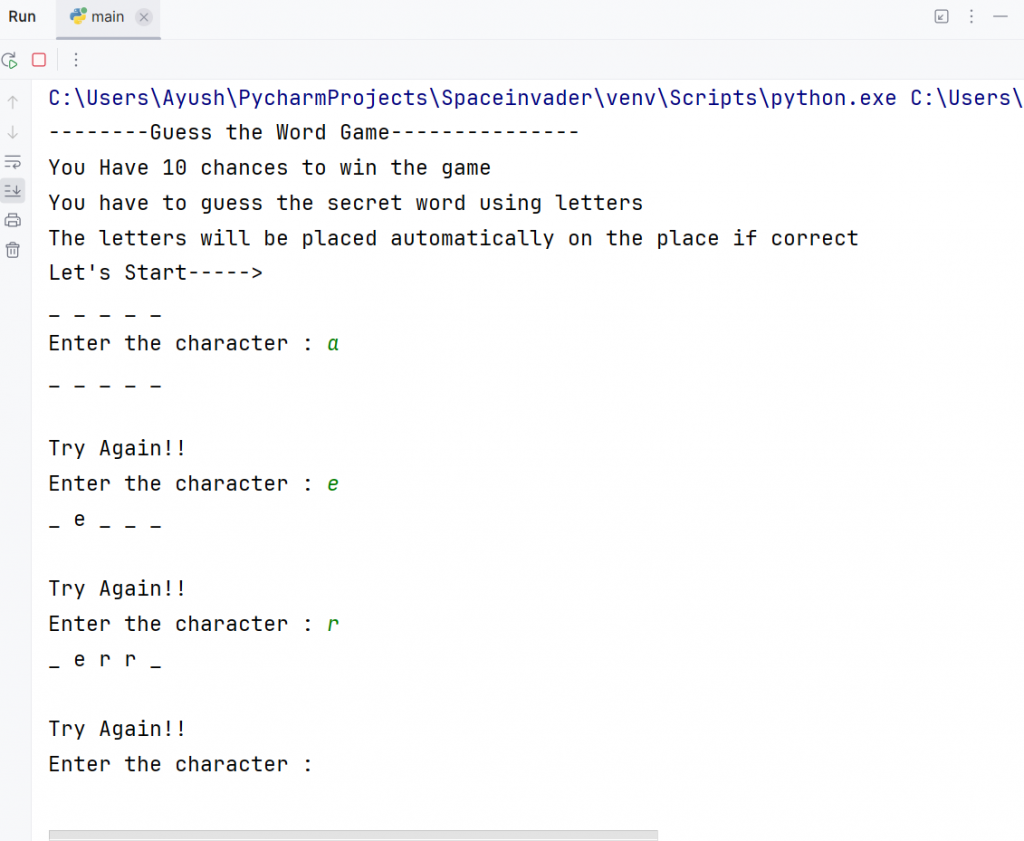
You are reading——-Python: Create Guess the Word game in 6 steps
Step 6: What if the user used all the chances and failed?
else:
print('You Lose the Game')
print('----------------------------------------------')
If the user fails to guess all the alphabets in the given 10 chances, the user has lost the game. The loop will be finished and jump to the else section of the loop and display the message “You lose the game”.
If you want to play this game on your own, copy these Python code blocks in a step-by-step manner in your Python editor and run. You are not required to download the random module. Just paste this code and run the program.
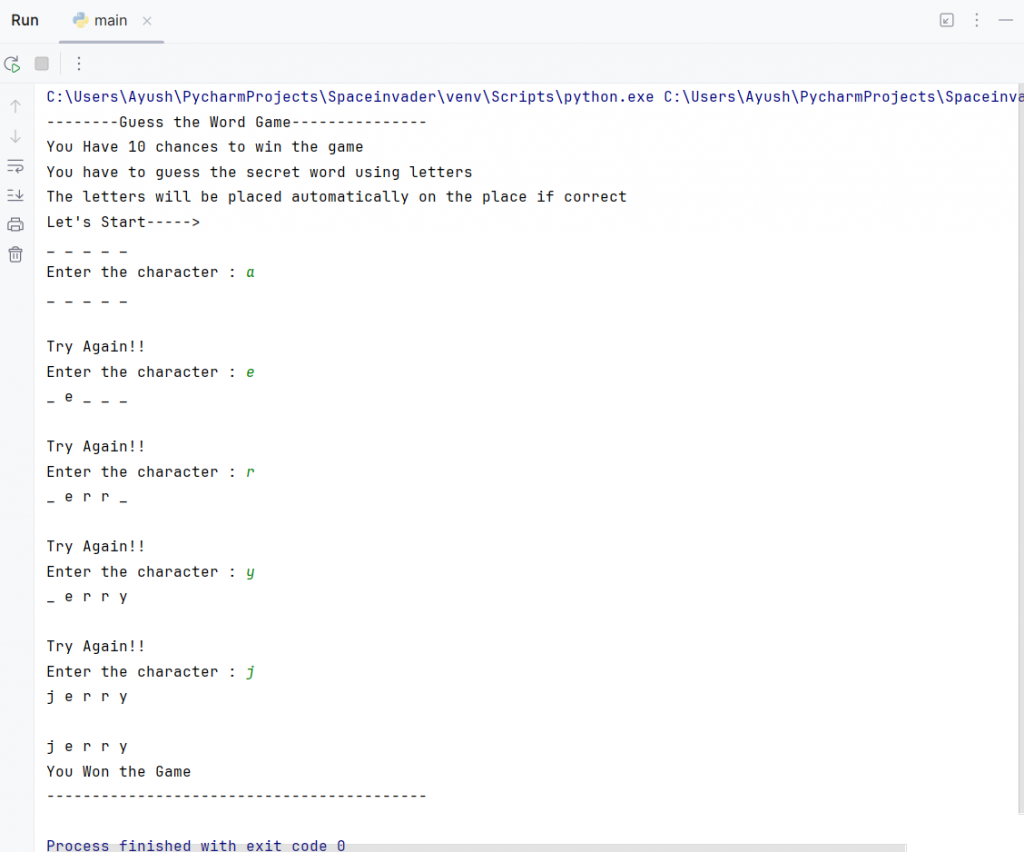
You are reading——-Python: Create Guess the Word game in 6 steps
Python programming language is also used for data science and if you wish to learn more about data science or want to curve your career in the data science field feel free to join our free workshop on Masters in Data Science with PowerBI, where you will get to know how exactly the data science field works and why companies are ready to pay handsome salaries in this field.
In this workshop, you will get to know each tool and technology from scratch that will make you skillfully eligible for any data science profile.
To join this workshop, register yourself on consoleflare and we will call you back.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,