Why file handling? When we create a program and run it, the console screen displays the output but the console screen has a volatile memory like RAM which means it has limited memory to accumulate a huge amount of data. So to achieve this, the console screen removes the old data and shows a new one which causes the loss of data.
To solve this problem we use the concept of file handling in Python which helps us store ample amounts of data for a long time. In the same way, we are going to create a project that will create a contacts book in a notepad file using the concept of file handling by receiving the data through a Python program.
We also recommend you read this blog on file handling for a better understanding of this project:
5 Essential Methods For File Handling In Python
Step 1: Creating functions for file handling project
In this step, we will start by creating functions for all the tasks happening in this project. In this function (display_menu()), we are printing all the operations that the user can perform.
def display_menu():
print("\n----- Contact Management System -----")
print("1. View Contacts")
print("2. Add Contact")
print("3. Search Contact")
print("4. Delete Contact")
print("5. Exit")
This function is displayed again and again on the screen and the user comes to know about the availability of operations one can perform.
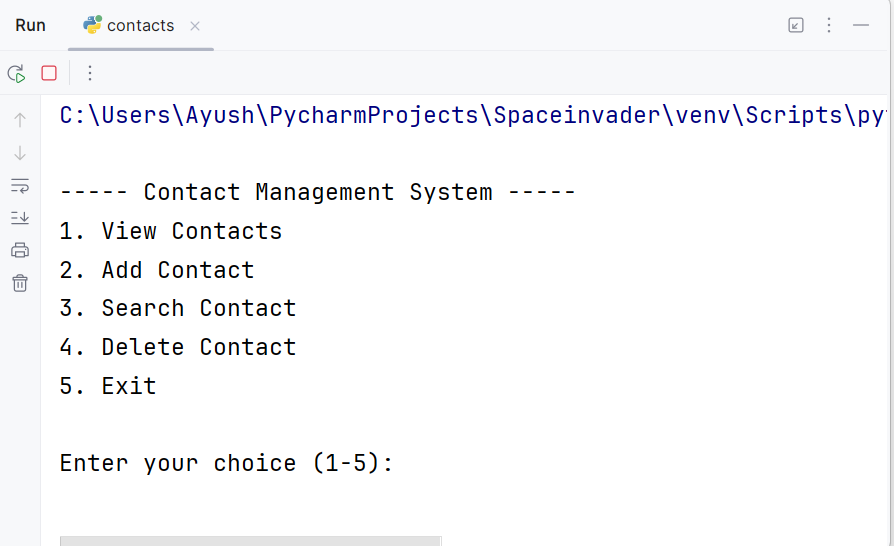
You are reading——-File Handling: Create your contacts book in a notepad
Step 2:
This function (view_contacts()) is created, if the user wants to see all the contacts at once.
def view_contacts():
with open("contacts.txt", "r") as file:
contacts = file.readlines()
if contacts:
print("\n----- Contacts -----")
for idx, contact in enumerate(contacts, start=1):
print(f"{idx}. {contact.strip()}")
else:
print("\nNo contacts available.")
In this function, firstly we read the content of the file with the readlines() method and store it in the contacts variable(data type list). After that, we check if the contacts variable is filled with data, if there is data we use a for loop and unpack the contacts variable into the idx variable(index number) and show the contacts stored. If the variable is empty, the program will jump on else and display the message “No contacts available”.
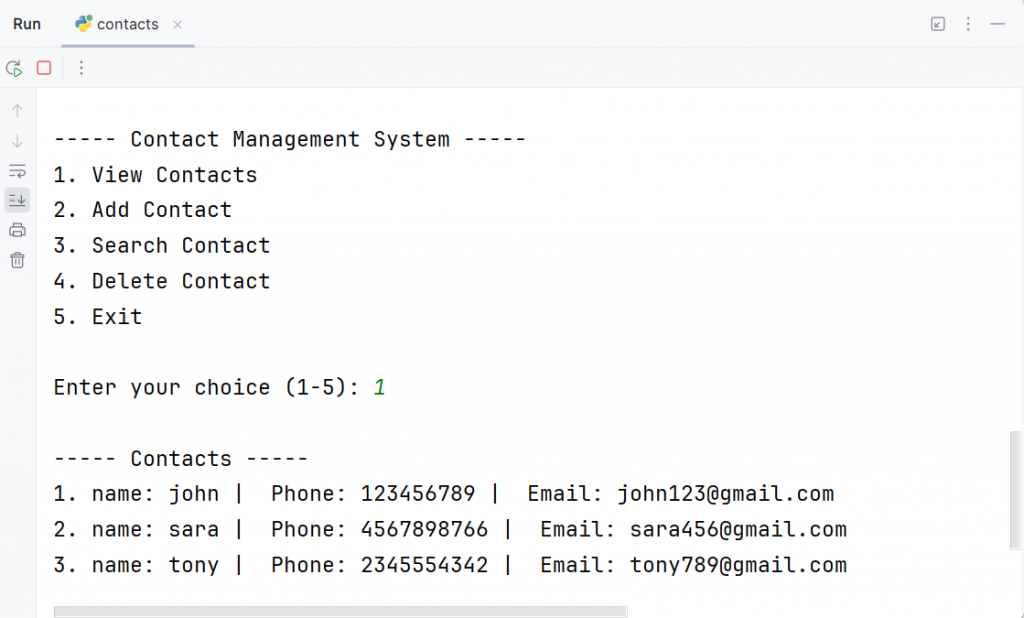
You are reading——-File Handling: Create your contacts book in a notepad
Step 3:
This function (add_contact()) is created, if the user wants to add a contact into the file.
def add_contact():
name = input("\nEnter the contact name: ")
phone = input("Enter the contact phone number: ")
email = input("Enter the contact email address: ")
contact_info = f"name: {name} | Phone: {phone} | Email: {email}\n"
with open("contacts.txt", "a") as file:
file.write(contact_info)
print(f"Contact '{name}' added successfully.")
In this function, we have used three variables name, phone, and email to receive data from the user, and later on we created one more variable that is contact_info which stores the variables name, phone, and email in the structured formatted string. Then we opened the file with access mode ‘a’ to append contact info into the file and display the message that the particular “contact added successfully”.
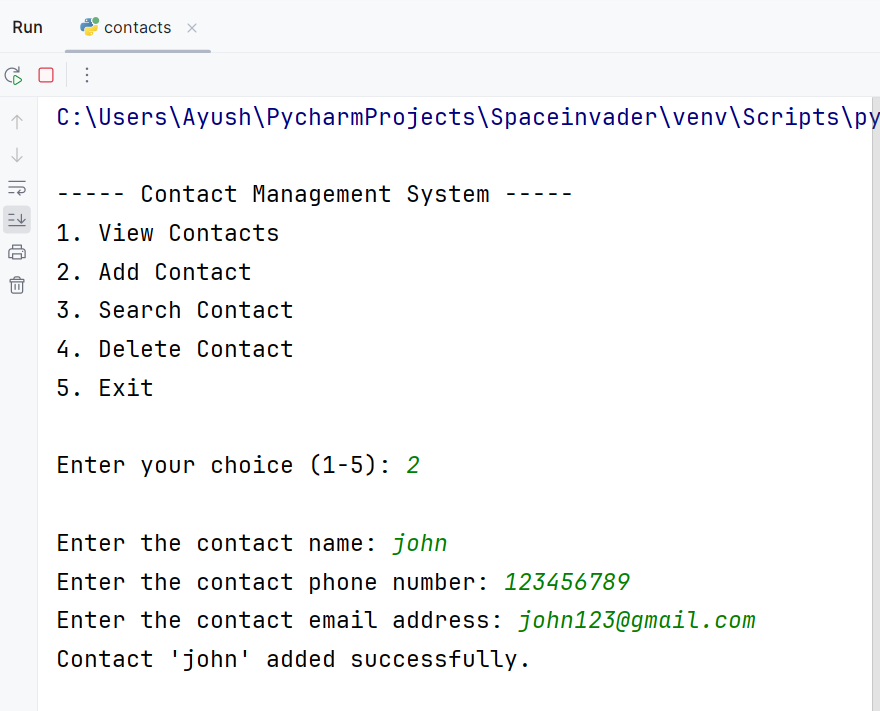
You are reading——-File Handling: Create your contacts book in a notepad
Step 4:
This function (search_contact()) is created, if the user wants to search for a contact in the file.
def search_contact():
keyword = input("\nEnter a keyword to search for a contact: ")
with open("contacts.txt", "r") as file:
contacts = file.readlines()
matching_contacts = [contact.strip() for contact in contacts if keyword.lower() in contact.lower()]
if matching_contacts:
print("\n----- Matching Contacts -----")
for idx, contact in enumerate(matching_contacts, start=1):
print(f"{idx}. {contact}")
else:
print("\nNo matching contacts found.")
In this function, we have created a variable keyword in which the user inputs the name, phone number, or email. After that, we open the file with access mode ‘r’ (read from a file) and store the contents of the file in the contacts variable. Later on, we use list comprehension to look for the keyword in the items of the contacts and store it in a variable matching contact. After that, if the contact is present it will get stored in the variable in the list data type and then we unpack that list with the help of the loop and enumerate function otherwise display the message “No matching contact found”.
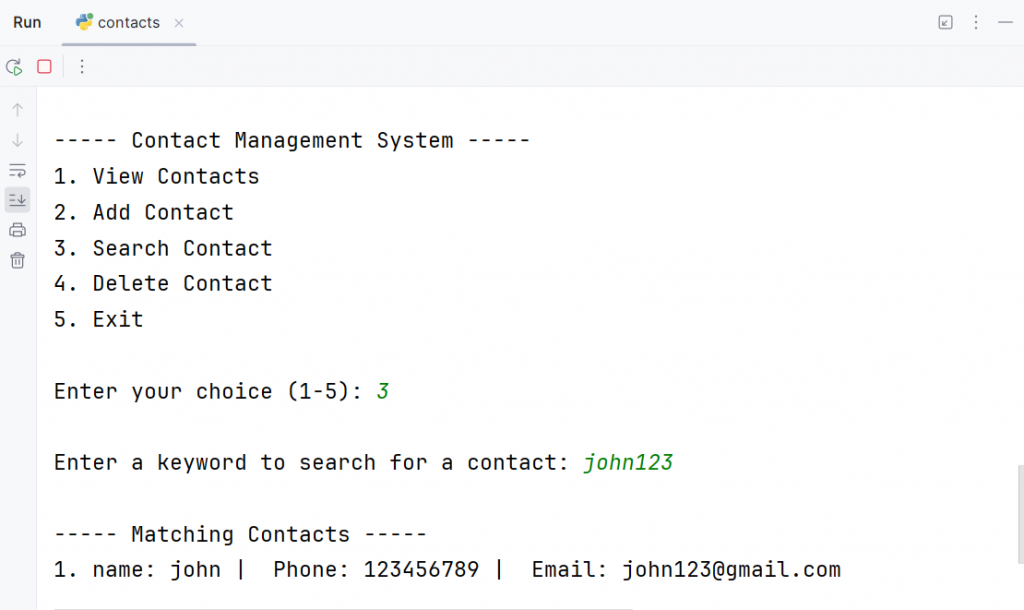
You are reading——-File Handling: Create your contacts book in a notepad
Step 5:
This function (delete_contact()) is created, if the user wants to delete a contact from the file.
def delete_contact():
view_contacts()
sr_number = int(input("\nEnter the contact number to delete: "))
with open("contacts.txt", "r") as file:
contacts = file.readlines()
if 1 <= sr_number <= len(contacts):
deleted_contact = contacts.pop(contact_number - 1)
with open("contacts.txt", "w") as file:
file.writelines(contacts)
print(f"Contact '{deleted_contact.strip()}' deleted successfully.")
else:
print("Invalid contact number. Please try again.")
In this function, firstly we call the view_contacts() function so that we can see all the contacts and then choose whichever contact the user has to delete. After that, we create a variable sr_num in which we receive the serial number of the contact the user wants to delete. We open the file again with access mode as ‘r’ and store the content of the file in the contacts variable. Later on, we used a conditional statement to check if the serial number the user has inputted exists in the contact variable or not. If it exists we used the pop() method to delete the contact.
You are reading——-File Handling: Create your contacts book in a notepad
After the contacts get deleted, we open the file with access mode ‘w’ which we use to overwrite a file and store the contacts in the file otherwise the program will jump to the else part and display the message “Invalid contact number. Please try again”.
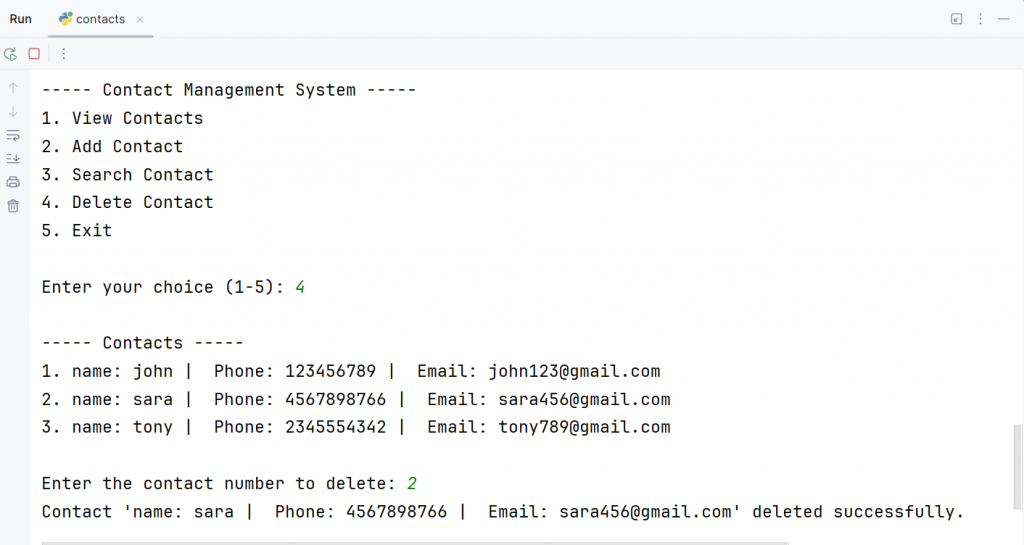
In the end, save this file by the name of contact_functions.py
You are reading——-File Handling: Create your contacts book in a notepad
For more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
After creating these functions in a file, now is the time to create a user interface that interacts with the user and performs actions as per the user’s requirement.
Step 1: Create a user interface for the file-handling project
We need to import functions from the contact_functions file and also import the os module
from contact_functions import *
import os
if os.path.isfile("contacts.txt"):
pass
else:
f=open('contacts.txt','x')
We have used a conditional statement which will use os.path.isfile() method to check if the file “contacts.txt” exists or not. If the file already exists means the condition is true and we have used the pass keyword to ignore the scenario and move on but if the file does not exist and the condition goes wrong, it will jump to the else part and a file by the name of contacts.txt is created.
You are reading——-File Handling: Create your contacts book in a notepad
Step 2:
Now we will create an infinite while loop with the condition True which will go on and on.
while True:
display_menu()
choice = input("\nEnter your choice (1-5): ")
if choice == "1":
view_contacts()
elif choice == "2":
add_contact()
elif choice == "3":
search_contact()
elif choice == "4":
delete_contact()
elif choice == "5":
print("Exiting the program. Goodbye!")
break
else:
print("Invalid choice. Please enter a number between 1 and 5.")
The purpose of this loop is to display the menu again and again by calling the function display_menu() and asking the user which operation the user wants to perform. If the user inputs “1”, the view_contacts() function will be called or if the user goes for options 2, 3, and 4 functions like add_contact(), search_contact(), and delete_contact() will be called and the operations will be performed as per the operations.
You are reading——-File Handling: Create your contacts book in a notepad
If you wish to learn more about data science or want to curve your career in the data science field feel free to join our free workshop on Masters in Data Science with PowerBI, where you will get to know how exactly the data science field works and why companies are ready to pay handsome salaries in this field.
In this workshop, you will get to know each tool and technology from scratch that will make you skillfully eligible for any data science profile.
To join this workshop, register yourself on consoleflare and we will call you back.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,