We all know that Python is a general-purpose programming language with lakhs of libraries that can help you in various projects. These libraries are created to make our tasks easy and efficient in all fields like if we talk about calculations we have numpy, if we talk about the analysis we have pandas, if we talk about graphs and charts we have seaborn and matplotlib.
In the same way, python also takes care of the developers who are highly interested in creating games on their own for which Python offers a library called pygame. This library offers us numerous functions to create a game on our own without having extra accessories and expenses.
In this blog, we will create a game of our own that is Space Invader in which you are a hero using a spacecraft and killing the aliens.
Step 1: Importing python libraries
To create this game we need to install and import python libraries like pygame, math, and random, and then import mixer from pygame.
import math
import random
import pygame
from pygame import mixer
Step 2:
Now we need to initialize the python library, create the screen with dimensions, create a background of the game, and introduce background music.
*Download these files in the same project files to run the game successfully.
# To intialize the pygame
pygame.init()
# To create the screen of the game.
screen = pygame.display.set_mode((800, 600))
# To use a background image in the game
background = pygame.image.load('background.png')
# To give sound effects in the background to the game.
mixer.music.load("background.wav")
mixer.music.play(-1)
# Caption/ Title
pygame.display.set_caption("Space Invader")
So this part of the code will help you set the screen, background image, background music and the title of the game.
For more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
Step 3:
In this step, we need to create variables for the player as well as enemies/aliens in the python code.
# Player
playerImg = pygame.image.load('player.png')
playerX = 370
playerY = 480
playerX_change = 0
# playerX and PlayerY are x and y cordinates of the spacecraft.
# Enemy
enemyImg = []
enemyX = []
enemyY = []
enemyX_change = []
enemyY_change = []
num_of_enemies = 6
# EnemyX and EnemyY are x and y cordinates of the enemies.
Step 4:
for i in range(num_of_enemies):
enemyImg.append(pygame.image.load('enemy.png'))
enemyX.append(random.randint(0, 736))
enemyY.append(random.randint(50, 150))
enemyX_change.append(4)
enemyY_change.append(40)
This python’s for loop will help us to create 6 enemies and they will appear on the screen from random positions also they will change their position as per the x and y coordinates which will look as moving and increase the difficulty of the game.
Step 5:
Now that we have created players and enemies, now is the time to create bullets. For that, we have to create variables for bullet and their positions in the python code.
# Bullet
# Ready - You can't see the bullet on the screen
# Fire - The bullet is currently moving
bulletImg = pygame.image.load('bullet.png')
bulletX = 0
bulletY = 480
bulletX_change = 0
bulletY_change = 10
bullet_state = "ready"
Step 6:
Now we have to create a variable for the score and set the fonts to display the score.
# Score
score_value = 0
font = pygame.font.Font('freesansbold.ttf', 32)
textX = 10
testY = 10
# Game Over
over_font = pygame.font.Font('freesansbold.ttf', 64)
Step 7:
Now we have to create Python functions for the events that are going to happen in the game like player, enemy, text, fire bullets, and collision.
def show_score(x, y):
score = font.render("Score: " + str(score_value), True, (255, 255, 255))
screen.blit(score, (x, y))
def game_over_text():
over_text = over_font.render("GAME OVER", True, (255, 255, 255))
screen.blit(over_text, (200, 250))
def player(x, y):
screen.blit(playerImg, (x, y))
def enemy(x, y, I):
screen.blit(enemyImg[i], (x, y))
def fire_bullet(x, y):
global bullet_state
bullet_state = "fire"
screen.blit(bulletImg, (x + 16, y + 10))
def isCollision(enemyX, enemyY, bulletX, bulletY):
distance = math.sqrt(math.pow(enemyX - bulletX, 2) + (math.pow(enemyY - bulletY, 2)))
if distance < 27:
return True
else:
return False
Step 8:
Now, let us start the game
# Game Loop
running = True
while running:
# RGB = Red, Green, Blue
screen.fill((0, 0, 0))
# Background Image
screen.blit(background, (0, 0))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
#This part of the python code is created to start the game and set the background image of the game. Later on, a for loop is used to receive to event happening in the game (buttons pressed and buttons released). For example if event.type== pygame.QUIT means the user wants to close the game, the user can click the close button#
# if keystroke is pressed check whether its right or left
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
playerX_change = -5
if event.key == pygame.K_RIGHT:
playerX_change = 5
if event.key == pygame.K_SPACE:
#if bullet_state is "ready":
bulletSound = mixer.Sound("laser.wav")
bulletSound.play()
# Get the current x cordinate of the spaceship
bulletX = playerX
fire_bullet(bulletX, bulletY)
if event.type == pygame.KEYUP:
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
playerX_change = 0
# 5 = 5 + -0.1 -> 5 = 5 - 0.1
# 5 = 5 + 0.1
playerX += playerX_change
if playerX <= 0:
playerX = 0
elif playerX >= 736:
playerX = 736
# This part of the python code is created to track which key is pressed or released and perform actions according to it for example if the left arrow is pressed move the player left or if the right arrow is pressed move the player to the right and if the space key is pressed then make the sound of shooting and fire bullet and the the arrow keys are released, stop the player at that point.
If the player goes to the extreme left or right side of the screen it must stop or it will leave the screen.#
# Enemy Movement
for i in range(num_of_enemies):
# Game Over
if enemyY[i] > 440:
for j in range(num_of_enemies):
enemyY[j] = 2000
game_over_text()
break
enemyX[i] += enemyX_change[i]
if enemyX[i] <= 0:
enemyX_change[i] = 4
enemyY[i] += enemyY_change[i]
elif enemyX[i] >= 736:
enemyX_change[i] = -4
enemyY[i] += enemyY_change[i]
# In this part of python code we have created a scenarion that if the enemy crosses the player the game will be over as well as we are also controlling the movements of the enemies in the x and y cordinates #
# Collision
collision = isCollision(enemyX[i], enemyY[i], bulletX, bulletY)
if collision:
explosionSound = mixer.Sound("explosion.wav")
explosionSound.play()
bulletY = 480
bullet_state = "ready"
score_value += 1
enemyX[i] = random.randint(0, 736)
enemyY[i] = random.randint(50, 150)
enemy(enemyX[i], enemyY[i], i)
# In this code of python we have created the steps about what must happen when the bullet touches the enemy. For that we have created the collision function. The sound of explosion will play. score will be updated and a new enemy will appear on the screen #
# Bullet Movement
if bulletY <= 0:
bulletY = 480
bullet_state = "ready"
if bullet_state is "fire":
fire_bullet(bulletX, bulletY)
bulletY -= bulletY_change
# In this part we have created the positioning of the bullet and its state of fire and when a bullet is fired the value of y cordinate will be decreased and then come to 0 which meas a new bullet is ready to get fired #
player(playerX, playerY)
show_score(textX, testY)
pygame.display.update()
# Atlast we are calling all these functions to show player and show score #
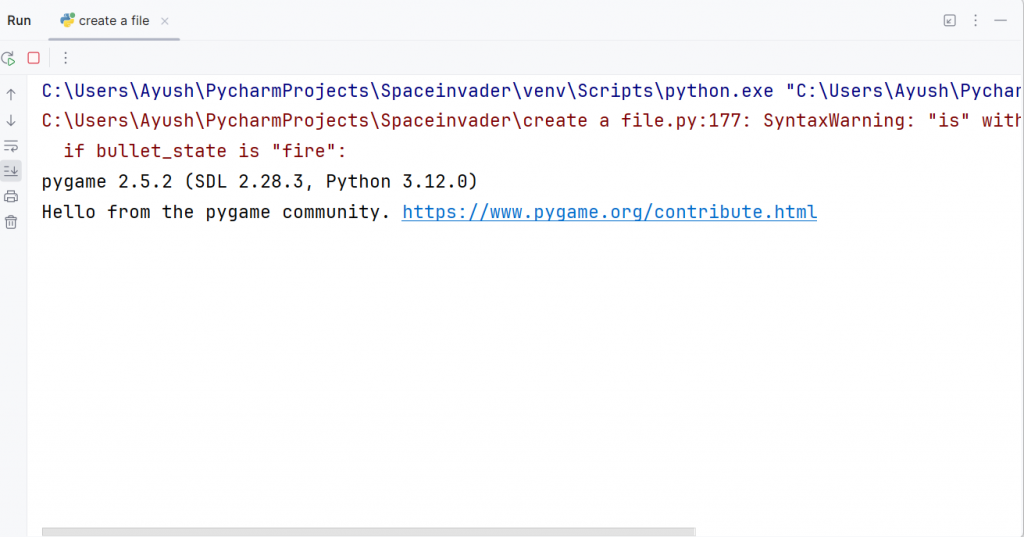
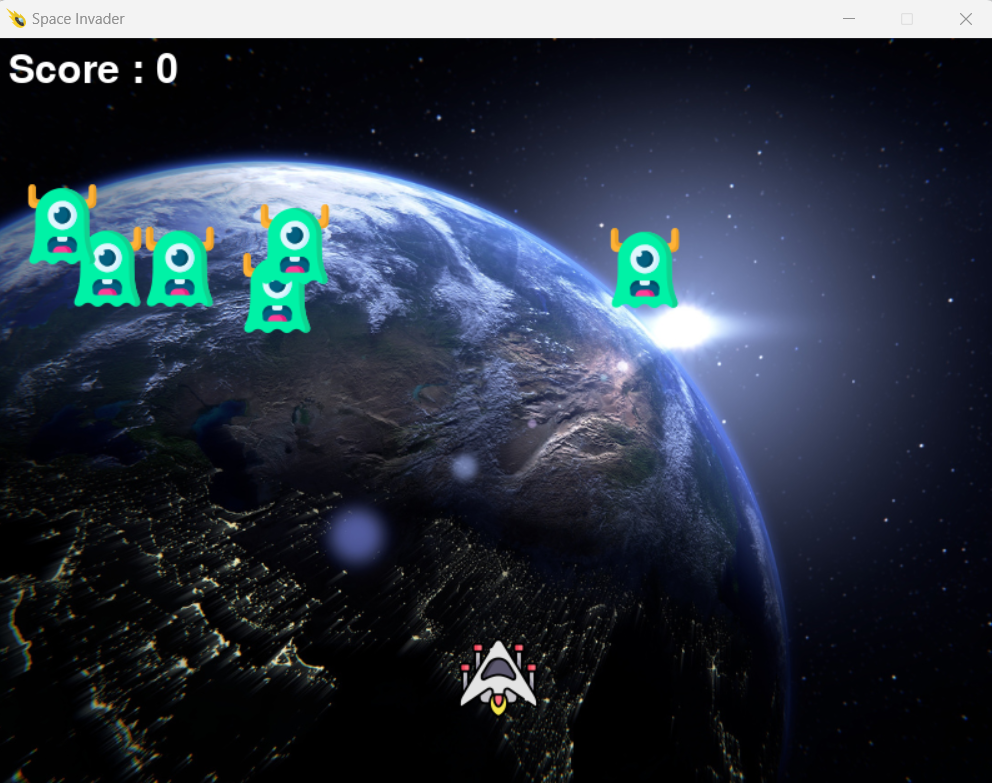
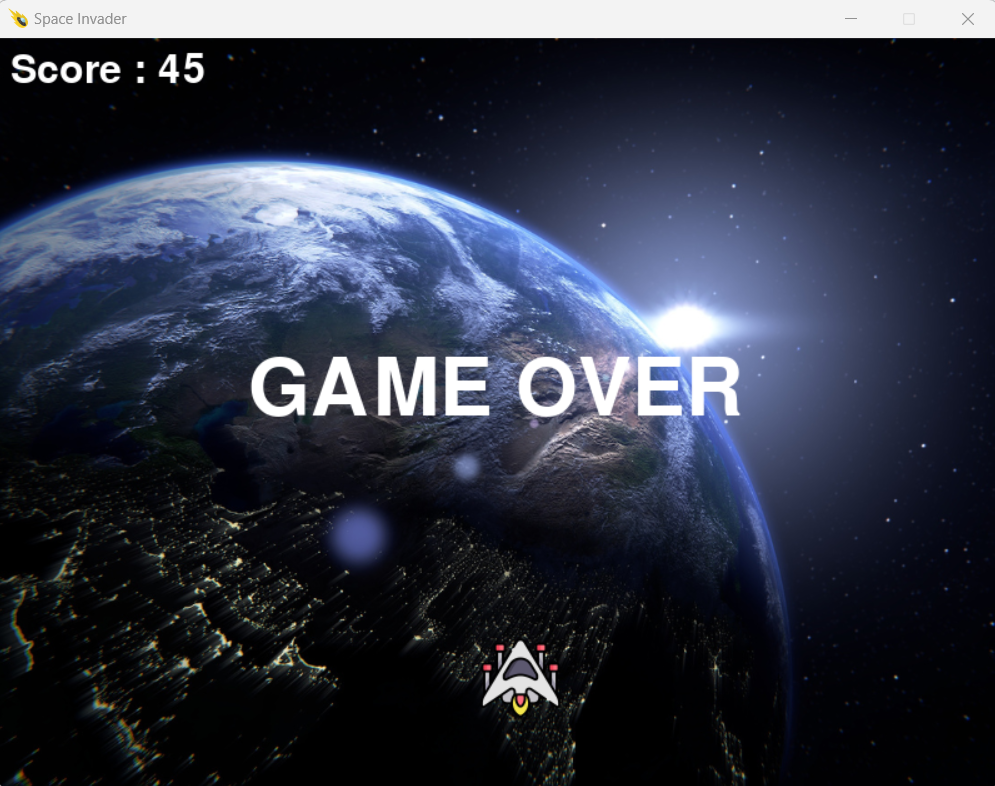
If you wish to learn more about data science or want to curve your career in the data science field feel free to join our free workshop on Masters in Data Science with PowerBI, where you will get to know how exactly the data science field works and why companies are ready to pay handsome salaries in this field.
In this workshop, you will get to know each tool and technology from scratch that will make you skillfully eligible for any data science profile.
To join this workshop, register yourself on consoleflare and we will call you back.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,