In today’s digital age, Python is one of the easiest languages to learn, work, and develop applications as per our requirements, with the help of a variety of libraries but in Python you can also develop projects that can use Notepad for handling data without using any library. In the same way in today’s times, managing personal finances is more important than ever.
A personal expense tracker can be a game-changer, helping you stay on top of your spending, save money, and make informed financial decisions. This blog will walk you through creating a simple yet effective personal expense tracker using Python. Whether you’re a coding beginner or someone looking to polish your Python skills, this project is for you. And if you’re inspired to dive deeper into the world of data science and analytics, we’ll show you how this project can be your gateway to a rewarding career in data science.
Why Build a Python-Based Personal Expense Tracker?
Before we dive into the code, let’s briefly discuss why building a personal expense tracker is a great project:
- Easy to create: Python has an OS module that has a collection of various functions and methods, that help you to interact with the operating system to work on notepad files.
- Practical Use: A personal expense tracker is a tool you can use daily. It helps you keep an eye on your spending, identify patterns, and make smarter financial decisions.
- Hands-On Learning: This project is a perfect way to practice Python, especially if you’re learning how to work with files, strings, and functions.
- Foundation for Data Science: Understanding how to handle data, even in a simple text file, is a fundamental skill in data science. This project lays the groundwork for more advanced data analysis tasks.
The Code Breakdown
Let’s break down the code step by step, explaining each function and its role in the overall program. By the end of this section, you’ll have a clear understanding of how the functions of a personal expense tracker work and how you can expand it to suit your needs:
1. Initializing the Expense Tracker: In this part, we will create a function that will check if the file exists otherwise it will create the file and store the heading of the data.
import os
def initialize_file():
if not os.path.exists('expenses.txt'):
with open('expenses.txt','w') as file:
file.write('Date, Amount,Category,Description\n')
Python2. Adding an Expense: In this function, we will open the file with access mode ‘a’ and code for accepting data, amount, category, and description as arguments store the data in the file, and display the message for expense added.
def add_expense(date, amount, category, description):
with open('expenses.txt', 'a') as file:
file.write(f'{date},{amount},{category},{description}\n')
print('Expense added')
Python3. Viewing All Expenses: In this function, we will open the file in read mode, access the content of the file in the list data type, and print the content of the file line by line using a for loop.
def view_expenses():
with open('expenses.txt', 'r') as file:
lines = file.readlines()
print(lines[0]) # Print the header
for line in lines[1:]:
print(line)
Python4. Filtering Expenses: This function will be responsible for filtering the expenses on behalf of either date or category as per the user’s requirement. This function will have two arguments. First is filter by(date or category) and second is filter value(date or category name) It will print the data of that particular date or category ignoring the other data.
def filter_expenses(filter_by, filter_value):
with open('expenses.txt', 'r') as file:
lines = file.readlines()
print(lines[0]) # Print the header
for line in lines[1:]:
data = line.split(',')
if filter_by == 'date' and filter_value == data[0]:
print(line)
elif filter_by == 'category' and filter_value == data[2]:
print(line)
Python5. Deleting an Expense: This function will allow the user to delete a particular entry of expense. This function will receive data, amount, category, and description as arguments. It will read the file first to access the content of the file then it will re-open the file again with access mode ‘w’ to overwrite the new data into the file(after deleting the expense), and display the message expense deleted.
def delete_expense(date, amount, category, description):
lines = []
with open('expenses.txt', 'r') as file:
lines = file.readlines()
with open('expenses.txt', 'w') as file:
for line in lines:
if line.strip() != f'{date},{amount},{category},{description}':
file.write(line)
print('Expense deleted')
Python6. Generating a Monthly Summary: This function will summarize all the expenses that happened in the current month. It will show the total expenses of the current month and then category-wise total expenses.
import datetime
def monthly_summary():
current_month = datetime.datetime.now().strftime('%Y-%m')
total_expense = 0.0
category_expense = {}
with open('expenses.txt', 'r') as file:
lines = file.readlines()
for line in lines:
data = line.strip().split(',')
if data[0].startswith(current_month):
amount = float(data[1])
category = data[2]
total_expense += amount
if category in category_expense:
category_expense[category] += amount
else:
category_expense[category] = amount
print(f'Total expense for {current_month}: {total_expense}')
for category, amount in category_expense.items():
print(f'{category}: {amount}')
PythonSave all these functions in a file so that all these functions can be imported into another file.
For more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
Now we need to create a main file that will import all the functions and create a menu for the user that will interact with the user and the program will follow the instructions as per the user’s requirements.
7. The Main function: This function will be called every time the program runs. This function will be responsible for displaying the menus, taking input from the user, and calling the functions as per the user’s requirements.
def main():
initialize_file()
while True:
print('1. Add Expense')
print('2. View Expense')
print('3. Filter Expense')
print('4. Delete Expense')
print('5. Monthly Summary')
print('6. Exit')
print()
choice = input('Select any one:')
if choice == '1':
date = input('Enter date(yyyy-mm-dd):')
amount = input('Enter amount:')
category = input('Enter category:')
description = input('Enter description:')
add_expense(date, amount, category, description)
print()
elif choice == '2':
view_expenses()
print()
elif choice == '3':
filter_by = input('Filter by(date/category):')
filter_value = input(f'enter {filter_by}:')
filter_expenses(filter_by, filter_value)
print()
elif choice == '4':
date = input('Enter date(yyyy-mm-dd):')
amount = input('Enter amount:')
category = input('Enter category:')
description = input('Enter description:')
delete_expense(date, amount, category, description)
print()
elif choice == '5':
monthly_summary()
elif choice == '6':
print('Exit from program')
break
else:
print('Invalid option')
if __name__ == '__main__':
main()
Python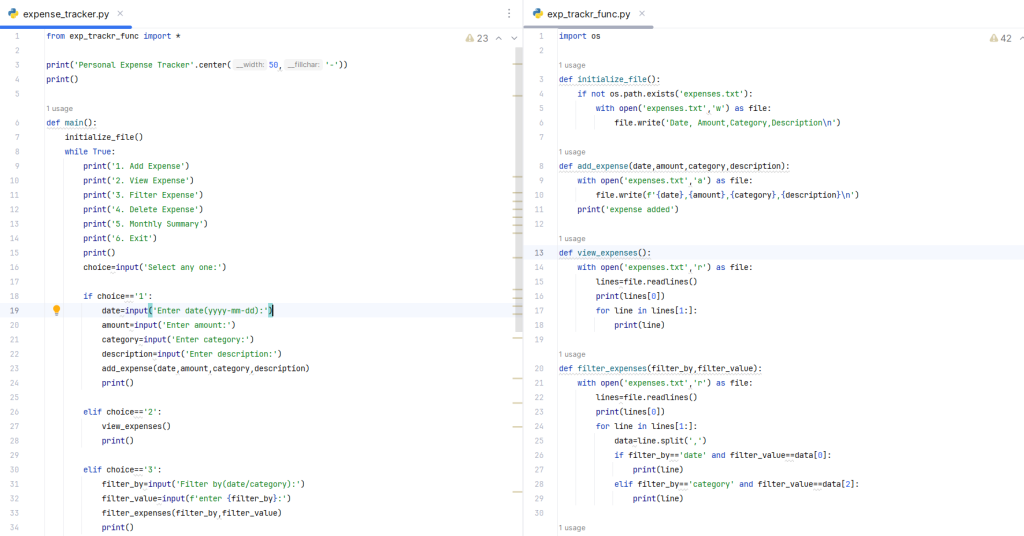
Expanding the Project: A Path to Data Science through Python: Now that we’ve built a basic personal expense tracker, you might be wondering, “What’s next?” The beauty of this project is its potential for expansion. Here are some ideas:
1. Data Analytics: You can use libraries like Pandas and Numpy for data analysis and statistical calculations.
2. Data Visualization: Use libraries like Matplotlib or Seaborn to create graphs that visualize your spending patterns.
3. Database Integration: Instead of storing data in a text file, you could use a database like SQLite or MySQL. This will introduce you to SQL, a crucial skill for data analysts.
Why Learn Data Science?
Data science is one of the most demanding skills in today’s job market. By learning data science, you can unlock countless opportunities in various industries. The skills you acquire can be applied to a wide range of tasks, from analyzing financial data to improving business operations.
Read more about DATA SCIENCE
Here’s how a data science course can benefit you:
High Demand: The demand for data scientists continues to grow as organizations increasingly rely on data-driven decision-making.
Diverse Career Paths: Data science skills are applicable in numerous fields, including finance, healthcare, marketing, and technology.
Lucrative Salaries: Data scientists are among the highest-paid professionals in the tech industry.
Continuous Learning: Data science is a field that constantly evolves, offering endless opportunities for growth and learning.
If you’re ready to embark on a rewarding career in data science, consider enrolling in a comprehensive course that focuses on Python.
At ConsoleFlare, we offer tailored courses that provide hands-on experience and in-depth knowledge to help you master Python and excel in your data science journey. Join us and take the first step towards becoming a data science expert with Python at your fingertips.
Register yourself with ConsoleFlare for our free workshop on data science. In this workshop, you will get to know each tool and technology of data analysis from scratch that will make you skillfully eligible for any data science profile.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,
Register yourself & we will help you switch your career to Data Science in just 6 months.
The complete code:
import os
def initialize_file():
if not os.path.exists('expenses.txt'):
with open('expenses.txt','w') as file:
file.write('Date, Amount,Category,Description\n')
def add_expense(date,amount,category,description):
with open('expenses.txt','a') as file:
file.write(f'{date},{amount},{category},{description}\n')
print('expense added')
def view_expenses():
with open('expenses.txt','r') as file:
lines=file.readlines()
print(lines[0])
for line in lines[1:]:
print(line)
def filter_expenses(filter_by,filter_value):
with open('expenses.txt','r') as file:
lines=file.readlines()
print(lines[0])
for line in lines[1:]:
data=line.split(',')
if filter_by=='date' and filter_value==data[0]:
print(line)
elif filter_by=='category' and filter_value==data[2]:
print(line)
def delete_expense(date,amount,category,description):
lines=[]
with open('expenses.txt','r') as file:
lines=file.readlines()
with open('expenses.txt','w') as file:
for line in lines:
if line.strip() != f'{date},{amount},{category},{description}':
file.write(line)
print('expense deleted')
import datetime
def monthly_summary():
current_month=datetime.datetime.now().strftime('%Y-%m')
total_expense=0.0
category_expense={}
with open('expenses.txt','r') as file:
lines=file.readlines()
for line in lines:
data=line.strip().split(',')
if data[0].startswith(current_month):
amount=float(data[1])
category=data[2]
total_expense+=amount
if category in category_expense:
category_expense[category] += amount
else:
category_expense[category]=amount
print(f'Total expense for {current_month}:{total_expense}')
for category,amount in category_expense.items():
print(f'{category}:{amount}')
# SAVE THE ABOVE FUNCTIONS IN A FILE AND SAVE AS exp_trackr_func.py
from exp_trackr_func import *
print('Personal Expense Tracker'.center(50,'-'))
print()
def main():
initialize_file()
while True:
print('1. Add Expense')
print('2. View Expense')
print('3. Filter Expense')
print('4. Delete Expense')
print('5. Monthly Summary')
print('6. Exit')
print()
choice=input('Select any one:')
if choice=='1':
date=input('Enter date(yyyy-mm-dd):')
amount=input('Enter amount:')
category=input('Enter category:')
description=input('Enter description:')
add_expense(date,amount,category,description)
print()
elif choice=='2':
view_expenses()
print()
elif choice=='3':
filter_by=input('Filter by(date/category):')
filter_value=input(f'enter {filter_by}:')
filter_expenses(filter_by,filter_value)
print()
elif choice=='4':
date = input('Enter date(yyyy-mm-dd):')
amount = input('Enter amount:')
category = input('Enter category:')
description = input('Enter description:')
delete_expense(date,amount,category,description)
print()
elif choice=='5':
monthly_summary()
elif choice=='6':
print('Exit from program')
break
else:
print('Invalid option')
if __name__ == '__main__':
main()
Python