Python is an incredibly powerful and versatile programming language that has gained popularity for its simplicity and ease of use. Whether you’re managing a small business or building complex data-driven applications, Python provides the tools you need to get the job done. One of the best ways to sharpen your Python skills is by working on practical projects that solve real-world problems.
In this blog, we’ll explore an Employee Management System built with Python. This system allows you to perform essential tasks like adding, updating, deleting, and displaying employee records. We will cover this project in a step-by-step manner, understanding all the functions and the flow of the program.
Whether you’re a beginner looking to solidify your programming skills or an experienced developer seeking to refresh your knowledge, this blog will guide you through creating a simple yet effective Employee Management System in Python.
We will start the code by defining all the functions first:
1. Python Variable to handle data of all employees
We will create a variable list_of_employees that will be an empty list that will store the data of the employees in multiple dictionaries.
list_of_employees = []
Python2. Function to add employee
In this function, we will receive data on the employee’s ID, name, age, position, and salary from the user. After that, we will check if the ID, inputted by the user is unique or not, and then we will store all the data in a dictionary and add that dictionary to the list_of_employees.
def add_employee():
check=True
print('Enter Employee Details')
id=int(input('ID:'))
name=input('Name:')
age=input('Age:')
position=input('Position:')
salary=int(input('Salary:'))
# This portion will be responsible to check the uniqueness of the id.
if not list_of_employees:
check=True
else:
for employee in list_of_employees:
if id==employee['id']:
check=False
break
# This portion will create a dictionary of all the data and add that dictioanry into the list
if check==True:
employee={
'id':id,
'name':name,
'age':age,
'position':position,
'salary':salary
}
list_of_employees.append(employee)
print('Employee added successfully')
else:
print('ID already exists.')
Python3. Function to update employees:
This function will be responsible for taking the ID from the user, checking if the ID exists in the data, and then showing the options to update like name, position, and salary. The user will again select an option to update. The program will show the old data, receive new data, and update the data in the list_of_employees variable. If the ID is not present in the variable, it will print ’employee not found.
def update_employee():
id=int(input('Enter ID:'))
for employee in list_of_employees:
if id == employee['id']:
print('Which information you want to update:')
print('1. Name')
print('2. Position')
print('3. Salary')
ch=input('Select any one to update:')
if ch=='1':
print(f'Name:{employee['name']}')
employee['name']=input('Enter new name:')
elif ch=='2':
print(f'Position:{employee['position']}')
employee['position']=input('Enter new position:')
elif ch=='3':
print(f'Salary:{employee['salary']}')
employee['salary']=input('Enter new salary:')
else:
print('Invalid choice.')
print('employee detail updated successfully')
print()
return
print('Employee not found')
Python4. Function to delete employee: This function will receive the ID from the user, check if it exists or not, and delete the complete dictionary that holds all the data of that particular ID. If the ID is not present in the variable, it will print ’employee not found.
def delete_employee():
id = int(input('Enter ID:'))
for employee in list_of_employees:
if id == employee['id']:
list_of_employees.remove(employee)
print('Employee deleted successfully')
return
print('Employee not found')
Python5. Function to display employees: This function will help us to display the details of all the employees in a tabular format. It will check first for the data in the variable and then start printing the details otherwise it will show no employee to display.
def display_employees():
if len(list_of_employees)==0:
print('No employee to display')
return
print('ID \t\t Name \t\t Age \t\t Position \t\t Salary')
for employee in list_of_employees:
print(f'{employee['id']} \t\t {employee['name']} \t\t {employee['age']} \t\t {employee['position']} \t\t {employee['salary']}')
PythonSave all these functions in a Python file-like myfunc.py.
For more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
Integrating the functions in another Python file:
1. Importing the functions from myfunc.py: We need access to all the functions from the myfunc.py file.
from myfunc import *
Python2. Creating an infinity loop and printing all the options: Now we will create an infinity loop using while, print the title of the project, and the options available to the users.
while True:
print()
print("----Employee Management System-----")
print()
print("1. Add Employee")
print("2. Update Employee")
print("3. Delete Employee")
print("4. Display Employees")
print("5. Exit")
print()
Python3. Asking the user to select any one operation: In this part, we will ask the user to select any one option and perform the task as per the user by calling the function. The last option will be to exit from the loop. If the user selects an option other than 1-5, it will display an invalid option.
choice = input("Select anyone: ")
if choice == '1':
add_employee()
print()
elif choice == '2':
update_employee()
print()
elif choice == '3':
delete_employee()
print()
elif choice == '4':
display_employees()
print()
elif choice == '5':
print('Exit from program')
break
else:
print("Invalid Option.")
Python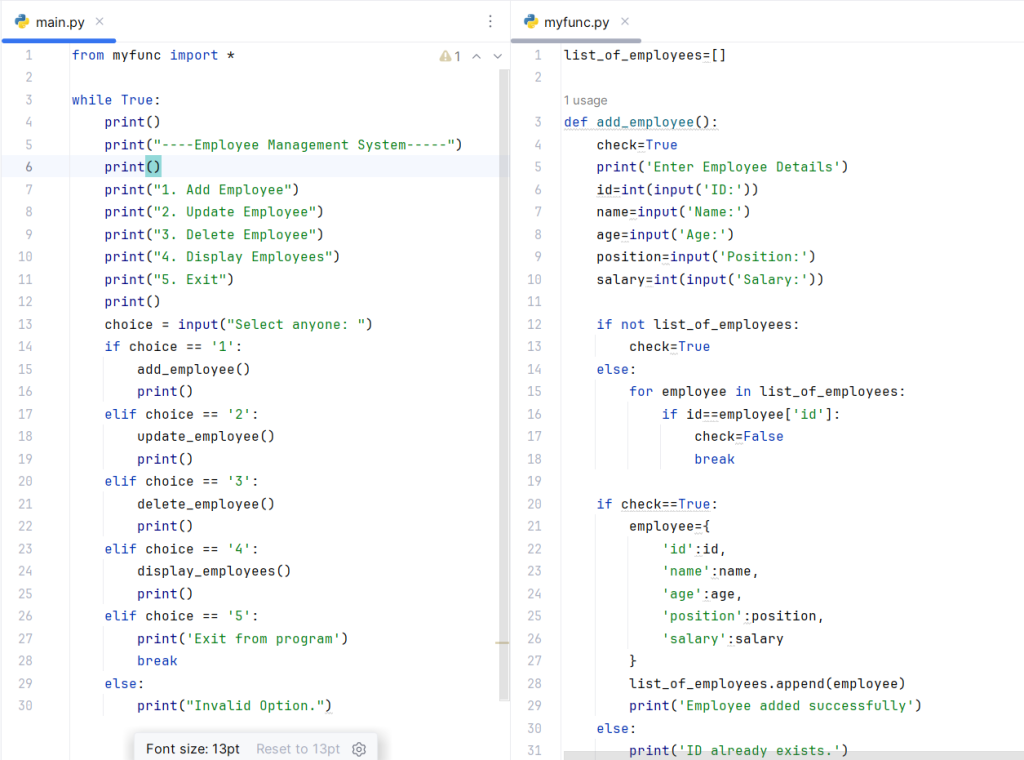
In this blog, we’ve walked through a simple yet effective employee management system built using Python. We started by understanding the basic structure of the script, explored each function in detail, and saw how the script integrates these functions to create a user-friendly system.
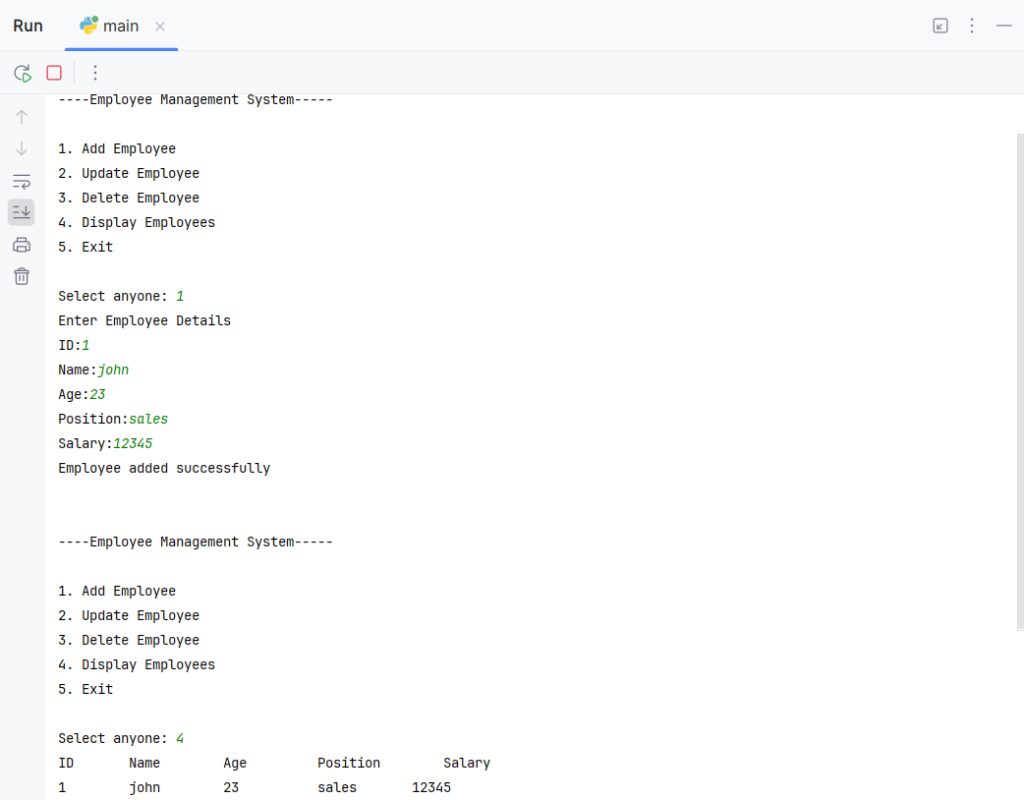
This script is a great starting point for anyone looking to learn Python, especially those interested in data science. It covers basic concepts like lists, dictionaries, loops, conditionals, and functions— all essential building blocks in Python programming.
Whether you’re managing a small team or learning Python for data science, understanding how to build such systems is crucial. As you grow your skills, you can extend this script to include more.
If you’re ready to embark on a rewarding career in data science, consider enrolling in a comprehensive course that focuses on Python.
At ConsoleFlare, we offer tailored courses that provide hands-on experience and in-depth knowledge to help you master Python and excel in your data science journey. Join us and take the first step towards becoming a data science expert with Python at your fingertips.
Register yourself with ConsoleFlare for our free workshop on data science. In this workshop, you will get to know each tool and technology of data analysis from scratch that will make you skillfully eligible for any data science profile.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,
Register yourself on consoleflare and we can help you switch your career to Data Science in just 6 months.
Happy coding, future data scientists!
The complete code:
#-----------------------------------------------------------------------------------------------#
# first file---- myfunc.py
list_of_employees=[]
def add_employee():
check=True
print('Enter Employee Details')
id=int(input('ID:'))
name=input('Name:')
age=input('Age:')
position=input('Position:')
salary=int(input('Salary:'))
if not list_of_employees:
check=True
else:
for employee in list_of_employees:
if id==employee['id']:
check=False
break
if check==True:
employee={
'id':id,
'name':name,
'age':age,
'position':position,
'salary':salary
}
list_of_employees.append(employee)
print('Employee added successfully')
else:
print('ID already exists.')
def update_employee():
id=int(input('Enter ID:'))
for employee in list_of_employees:
if id == employee['id']:
print('Which information you want to update:')
print('1. Name')
print('2. Position')
print('3. Salary')
ch=input('Select any one to update:')
if ch=='1':
print(f'Name:{employee['name']}')
employee['name']=input('Enter new name:')
elif ch=='2':
print(f'Position:{employee['position']}')
employee['position']=input('Enter new position:')
elif ch=='3':
print(f'Salary:{employee['salary']}')
employee['salary']=input('Enter new salary:')
else:
print('Invalid choice.')
print('employee detail updated successfully')
print()
return
print('Employee not found')
def delete_employee():
id = int(input('Enter ID:'))
for employee in list_of_employees:
if id == employee['id']:
list_of_employees.remove(employee)
print('Employee deleted successfully')
return
print('Employee not found')
def display_employees():
if len(list_of_employees)==0:
print('No employee to display')
return
print('ID \t\t Name \t\t Age \t\t Position \t\t Salary')
for employee in list_of_employees:
print(f'{employee['id']} \t\t {employee['name']} \t\t {employee['age']} \t\t {employee['position']} \t\t {employee['salary']}')
#--------------------------------------------------------------------------------------------#
# Second file
from myfunc import *
while True:
print()
print("----Employee Management System-----")
print()
print("1. Add Employee")
print("2. Update Employee")
print("3. Delete Employee")
print("4. Display Employees")
print("5. Exit")
print()
choice = input("Select anyone: ")
if choice == '1':
add_employee()
print()
elif choice == '2':
update_employee()
print()
elif choice == '3':
delete_employee()
print()
elif choice == '4':
display_employees()
print()
elif choice == '5':
print('Exit from program')
break
else:
print("Invalid Option.")
Python