In programming, very few tools are as versatile and powerful as regular expressions (regex). They allow you to search, match, and manipulate strings with great ease. In Python, the re
gex module provides the methods and functions to search, match, and manipulate strings. Whether you’re a new data scientist or a developer, having a strong knowledge of the regex module can improve your coding skills. In this blog, we will cover all the aspects of the regex module in a step-by-step manner but before proceeding let us understand,
What is Regex?
Regex, short for regular expressions, is a collection of characters that define a search pattern. It is commonly used for string searching, pattern matching, and string manipulation. Regex may seem confusing at first due to its syntax, but once you get the crux of it, you’ll find it an irreplaceable tool. But the question arises that exactly
Why do we use regex?
String manipulations and pattern searching or matching can be done in other ways too but the regex module helps us in doing these tasks a lot better way, faster, and easily.
Powerful Pattern Matching: The regex module allows you to create custom complex searches and patterns to match with strings.
String Manipulation: It helps us in performing string operations like substitutions and splitting easily.
Efficiency: The Regex module performs pattern search, match, and manipulation tasks efficiently in easy and fewer lines of code.
Let us get started with the regex module,
Importing: Regex is not a library that we need to download. Like other modules we have to just import it.
import re
PythonFunctions used in the Regex
- re.search(): It looks for the first appearance of the pattern within a string. It returns a match object if found, otherwise returns
None
.
import re
match = re.search(r'\d+', 'The year is 2024')
if match:
print("Found a number:", match.group())
else:
print(match)
# Output----- Found a number: 2024
Python2. re.match(): It looks for a match only at the beginning of the string. Returns a match object if found, otherwise returns None
.
match = re.match(r'\d+', '2024 is the year')
if match:
print("Found a number at the beginning:", match.group())
else:
print(match)
# Output----- Found a number at the beginning: 2024
Python3. re.findall(): It gives the result of all non-overlapping matches of a pattern in a string as a list.
numbers = re.findall(r'\d+', '2024 is the year with 12 months and 365 days')
print("Numbers found:", numbers)
# Output------ Numbers found: ['2024', '12', '365']
Python4. re.finditer(): It gives the result in the form of an iterator yielding match objects for all non-overlapping matches.
matches = re.finditer(r'\d+', '2024 is the year with 12 months and 365 days')
for match in matches:
print("Found number:", match.group())
# Output
# Found number: 2024
# Found number: 12
# Found number: 365
Python5. re.sub(): It replaces occurrences of a pattern with a replacement string in the entire string.
result = re.sub(r'\d+', 'NUMBER', '2024 is the year with 12 months 365 days')
print("After substitution:", result)
# Output----- After substitution: NUMBER is the year with NUMBER months NUMBER days
PythonFor more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
Components of Patterns in regex:
In regex, we use literal characters and symbols to create a pattern. Here are some fundamental components,
Literals: It means direct characters to match.
Metacharacters: It means symbols with special meanings like ‘.’ for any character, ‘^’ for the start of a string, and ‘$’ for the end of the string.
Character Classes: It defines a set of characters to match (e.g., [a-z]
for any lowercase letter).
Quantifiers: It specifies the number of occurrences (e.g., *
for 0 or more, +
for 1 or more, {n}
for exactly n).
Example Patterns
- Digits:
'\d
‘ - Word Characters:
'\w
‘ - Whitespace Characters: ‘
\s'
- Non-Digits:
'\D
‘ - Non-Word Characters:
'\W
‘ - Non-Whitespace Characters:
'\S
‘
Anchors: These are used to specify the position of the pattern within the string.
^: Start of the string
$: End of the string
\b: Word boundary
\B: Non-word boundary
Now we need to create groups and capture parts of the matching pattern. This is done with the help of parenthesis.
match = re.search(r'(\d{4})-(\d{2})-(\d{2})', 'Date: 2024-06-10')
if match:
print("Year:", match.group(1))
print("Month:", match.group(2))
print("Day:", match.group(3))
# Output------
# Year: 2024
# Month: 06
# Day: 10
PythonAdvances Features
Named Groups: It allows you to assign a name to a group for easier access.
match = re.search(r'(?P<year>\d{4})-(?P<month>\d{2})-(?P<day>\d{2})', 'Date: 2024-06-10')
if match:
print("Year:", match.group('year'))
print("Month:", match.group('month'))
print("Day:", match.group('day'))
# Output
# Year: 2024
# Month: 06
# Day: 10
PythonLookahead and Lookbehind: They allow you to assert a pattern without including it in the match.
Positive Lookahead:(?=...)
Negative Lookahead:(?!...)
Positive Lookbehind:(?<=...)
Negative Lookbehind:(?<!...)
# Positive lookahead example
match = re.search(r'\d+(?= dollars)', 'The price is 50 dollars')
if match:
print("Price:", match.group())
# Output--- Price: 50
PythonFlags: They help us to modify the behavior of regex. They can be passed as a second argument to functions like re.search(), re.match(), etc.
re.IGNORECASE (or re.I): Ignore the case.
re.MULTILINE (or re.M): Multi-line matching.
re.DOTALL (or re.S): Make .
match any character, including newlines.
match = re.search(r'test', 'This is a TEST', re.IGNORECASE)
if match:
print("Found:", match.group())
# output---- TEST (The case of the characters in test got ignored here)
PythonSome of the practical examples where regex helps you a lot.
- Email Validation: Validating an email address is a very common task that can be done easily with the help of regex.
pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'
emails = ['user@example.com', 'invalid-email', 'another.user@domain.co']
valid_emails = [email for email in emails if re.match(pattern, email)]
print("Valid emails:", valid_emails)
# Output--- Valid emails: ['user@example.com', 'another.user@domain.co']
Python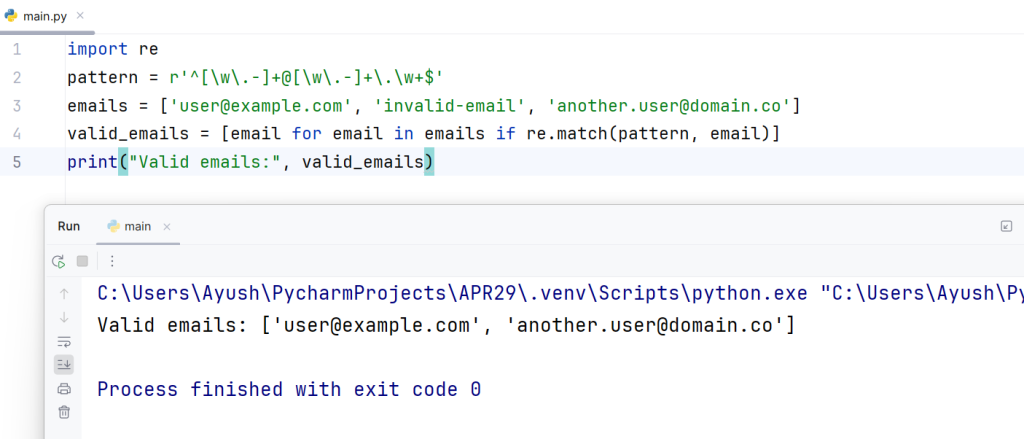
2. URL Extraction: Extracting URLs from a block of text helps us a lot in web scraping or data analysis.
text = 'Visit our website at https://www.example.com or follow us at http://www.example.org.'
urls = re.findall(r'https?://[^\s]+', text)
print("Found URLs:", urls)
# output---- Found URLs: ['https://www.example.com', 'http://www.example.org.']
Python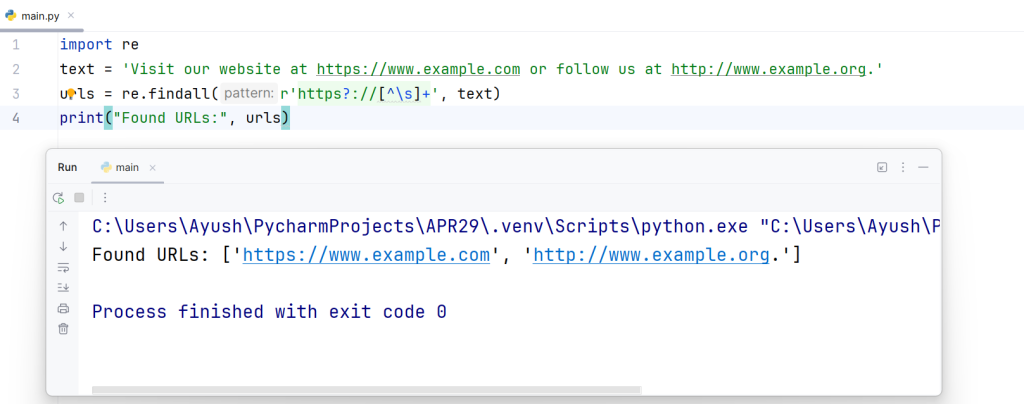
3. Password strength checker: You can use regex to check the strength of passwords by ensuring they contain a mix of characters.
pattern = r'^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$'
passwords = ['StrongPass1!', 'weakpass', '12345', 'Password!']
strong_passwords = [pwd for pwd in passwords if re.match(pattern, pwd)]
print("Strong passwords:", strong_passwords)
# Output--- Strong passwords: ['StrongPass1!']
Python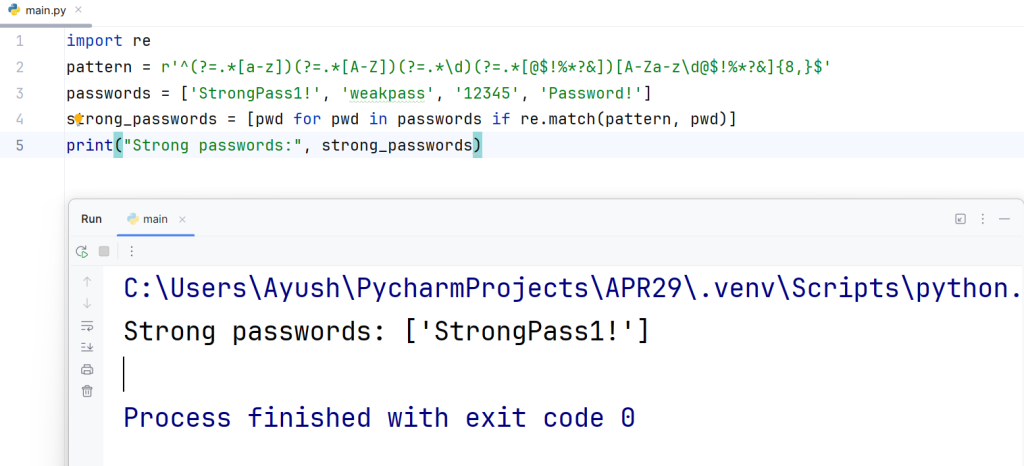
Conclusion
In the end, we can conclude that the re
gex module in Python is an incredibly powerful tool for anyone dealing with text processing and pattern matching. By mastering regex, you can perform complex searches, replacements, and manipulations with ease. While regex might seem intimidating at first, practice and experimentation will help you become more comfortable with its syntax and capabilities.
Regular expressions are not just a tool; they are a skill that can dramatically improve your efficiency and effectiveness as a data scientist. With the knowledge and examples provided in this guide, you should be well on your way to becoming proficient with Python’s re
module. Happy coding!
If you wish to learn data analysis and curve your career in the data science field, feel free to join our free workshop on Masters in Data Science with PowerBI, where you will get to know how exactly the data science field works and why companies are ready to pay handsome salaries in this field.
In this workshop, you will get to know each tool and technology of data analysis from scratch that will make you skillfully eligible for any data science profile.
To join this workshop, register yourself on consoleflare and we will call you back.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,