Working successfully with data and writing readable and performant code is what we are all striving for. A Python dictionary is a key-value map with unique keys. One part that is crucial in achieving that is selecting the ideal data structure for a given problem. A data structure is a particular way to organize data so that it can be efficiently stored, retrieved, or updated.
Python natively ships with various built-in data structures for example lists, tuples, sets, queues, or dictionaries just to mention a few. In this post, we will have a look at dictionaries. I try to cover a broad range from very basic usage for newbies up to advanced topics for pros. Let’s see if I can deliver on that.
What is a Python Dictionary?
The corresponding values can be of any type. In other programming languages, you might find terms like map, hashmap, associative array, or lookup table which all refer to the same concept.
So, what are dictionaries good for? They are good for accessing elements by key in a quick and readable way without having to search through a full dataset. This means you can achieve both performance gains and write more readable code using dictionaries. Now, what properties do they have?
Python Dictionary is changeable, or mutable if you want to be cocky. This means you can update, add, or remove key-value pairs at any time.
Furthermore, since Python dictionary 3.7 preserves insertion order. I.e., when you iterate over the elements of a dictionary, the elements will be traversed in the same order as they were added. If you use Python 3.6 or earlier, which I hope you don’t, you have to use an OrderedDict to guarantee the order of your dictionary.
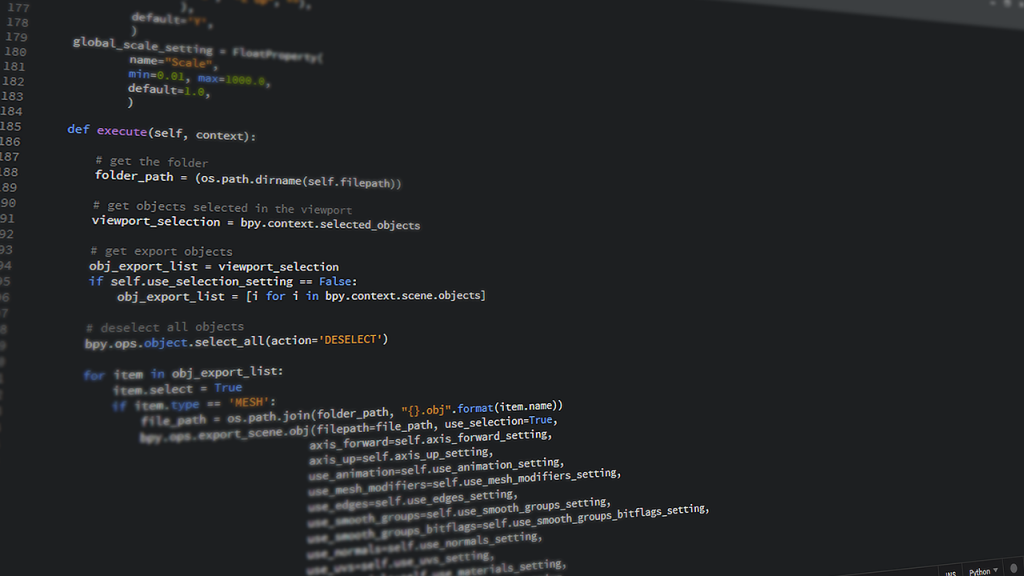
The last point I want to mention before we start looking into real code is that dictionary keys must be hashable. You also often read that keys must be immutable, but that is just because immutable types are also hashable. Examples of hashable types that you can use as keys are numbers or strings or tuples of numbers or strings. Mutable types that you cannot use as keys are for example lists or dictionaries. So you cannot use a dictionary as a key in a dictionary. As a side note, if you want to use an instance of some class as a key, you have to implement the magic function hash for that class.
There are two basic ways to create a Python dictionary. First, you can use curly braces and add comma-separatedkey: value
pairs to it. Second, you can use the dict
class and pass key-value pairs to the constructor. This is nice when your keys are strings and also proper Python names. If not, you would have to pass an iterable of tuples of the form (key, value)
to dict
. This is actually what you get in a very compact way when writing dict(zip(keys, values))
.
Another way to create a Python dictionary is by combining multiple dictionaries. How can you do that? You can do that by unpacking dictionaries with the **
operator and combining them by putting that in curly braces, see line 9. An alternative to that introduced in Python 3.9 is using the |
operator to join dictionaries.
With Python dictionaries, we can replace certain if-else statements and make our code less verbose and cleaner. Alternatively, we can define a dictionary that maps the warehouse name to the corresponding function.
Content Reference: Towards Data Science
In this post, we talked about what Python dictionaries are and how to work with them efficiently. To read more such articles, click here…