Streamlit is a popular framework for building interactive web applications with Python. In this blog post, we’ll introduce you to a Streamlit toolkit that empowers you to effortlessly create and scan QR codes using Python. With just a few lines of code, you’ll be generating and decoding QR codes like a pro.
But before proceeding let us understand what is QR first. QR stands for Quick Response. In a world where convenience is on the top, QR codes have emerged as a simple yet powerful tool for seamless information exchange whether you want to encode data for marketing campaigns, share contact information, or facilitate mobile payments, QR codes offer a simple and convenient solution.
Let us start with the code
Step 1: Setting Up the Environment (streamlit, pyqrcode, opencv-python, pyzbar)
Before starting the code, we need to set up the environment first. For that we need to download and import the following libraries like streamlit, pyqrcode, opencv, and pyzbar. You can also use pip for that.
import streamlit as st
import pyqrcode
from io import BytesIO
from PIL import Image
import cv2
from pyzbar import pyzbar
PythonStep 2: Generate QR Code
In this step, we will create a function that will generate QR for the data which will be inputted by the user.
The data inputted by the user will be in
def generate_qr_code(data):
"""
Generates a QR code image from the provided data.
Parameters:
data (str): The data to encode in the QR code.
Returns:
BytesIO: The QR code image in a BytesIO buffer.
"""
qr = pyqrcode.create(data)
buffer = BytesIO()
qr.png(buffer, scale=6)
buffer.seek(0)
return buffer
PythonStep 3: Decode QR Code
Next, we will create a function to decode QR codes from images. This is where OpenCV and pyzbar
come into play. We define a function decode_qr_code(frame)
that takes an image frame (in the form of a NumPy array) as input. Using OpenCV, we capture frames from the camera and pass them to pyzbar
for QR code decoding. The decoded data is returned as a list of strings
def decode_qr_code(frame):
"""
Decodes QR codes in the given image frame.
Parameters:
frame (numpy.ndarray): The image frame to scan for QR codes.
Returns:
list: A list of decoded QR code data.
"""
decoded_objects = pyzbar.decode(frame)
qr_codes = [obj.data.decode('utf-8') for obj in decoded_objects]
return qr_codes
PythonYou are reading Streamlit app for QR Toolkit in 6 simple steps
Step 4: Creating QR Codes in Real-Time
Here, we will code using streamlit functions and call the function “generate_qr_code” so that a qr code can be created instantly on behalf of the data inputted by the user. This function will create a QR image and also a download button will be active to download the QR in png format.
def create_qr_code():
st.title("QR Code Generator")
data = st.text_input("Enter the data to encode in the QR code") # input by user
if data:
qr_image = generate_qr_code(data) # function generate_qr_code with data as argument
img = Image.open(qr_image)
st.image(img, caption="Generated QR Code", use_column_width=False)
st.download_button(
label="Download QR Code",
data=qr_image,
file_name="qrcode.png",
mime="image/png"
)
PythonStep 5: Scanning and Decoding QR Codes in Real-Time
In this function, we will code to decode a QR scanned through your laptop camera. This function will be solely responsible for scanning a QR and also since we are making it a streamlit application we need to create 2 more functions to start and stop scanning which will be called as per requirement.
After scanning a QR frame by frame, we will call the function decode_qr_code which will decode the QR and all of this will happen in a loop which will be stopped after decoding the QR.
def scan_qr_code():
st.title("QR Code Scanner")
st.write("Click the button below to start the camera and scan a QR code.")
# Initialize session state for control buttons and decoded message
if "scanning" not in st.session_state:
st.session_state.scanning = False
if "decoded_message" not in st.session_state:
st.session_state.decoded_message = None
def start_scanning():
st.session_state.scanning = True
def stop_scanning():
st.session_state.scanning = False
if not st.session_state.scanning:
if st.button('Start Scanning', key='start'):
start_scanning()
if st.session_state.scanning:
cap = cv2.VideoCapture(0)
stframe = st.empty()
while st.session_state.scanning:
ret, frame = cap.read()
if not ret:
st.write("Failed to capture image.")
break
qr_codes = decode_qr_code(frame)
# Display the frame
stframe.image(frame, channels="BGR")
# Display the decoded QR codes if it's a new message
if qr_codes:
if st.session_state.decoded_message != qr_codes[0]:
st.session_state.decoded_message = qr_codes[0]
st.success(f"Decoded QR Code: {qr_codes[0]}")
break
if st.button('Stop Scanning', key='stop', on_click=stop_scanning):
cap.release()
cv2.destroyAllWindows()
PythonFor more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
Step 6: Putting It All Together– The Main program
Here we will use radio buttons with name like “Create QR” and “Scan QR” and the user has to choose any one option. As per the user’s choice the functions from above will be called.
st.title("QR Code Toolkit")
option = st.radio(
"Choose an option:",
("Create QR", "Scan QR")
)
if option == "Create QR":
create_qr_code()
elif option == "Scan QR":
scan_qr_code()
PythonAnd there you have it – a simple yet powerful QR code toolkit built with Python and Streamlit whether you’re a pro developer or a noob, you just have to follow the instructions step by step and Bingo! you have created your app.
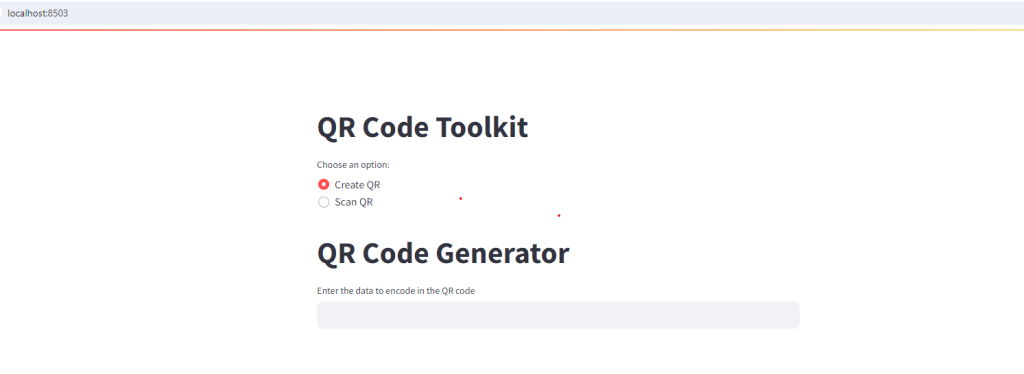
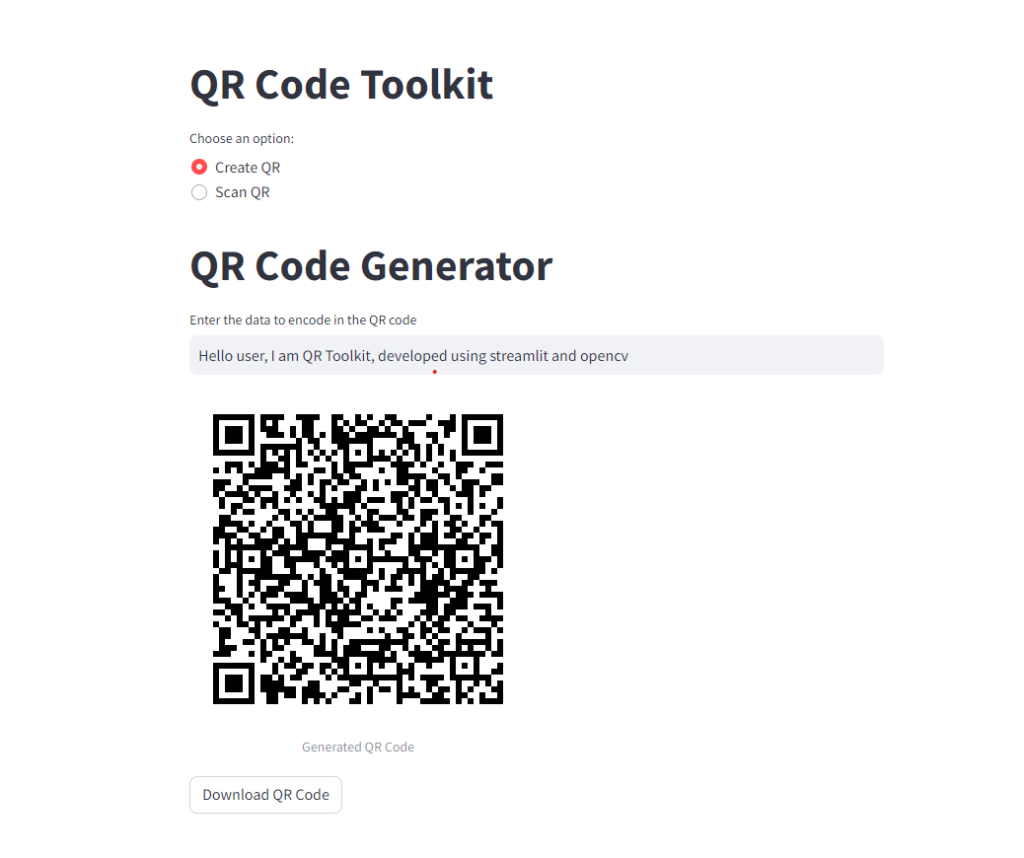
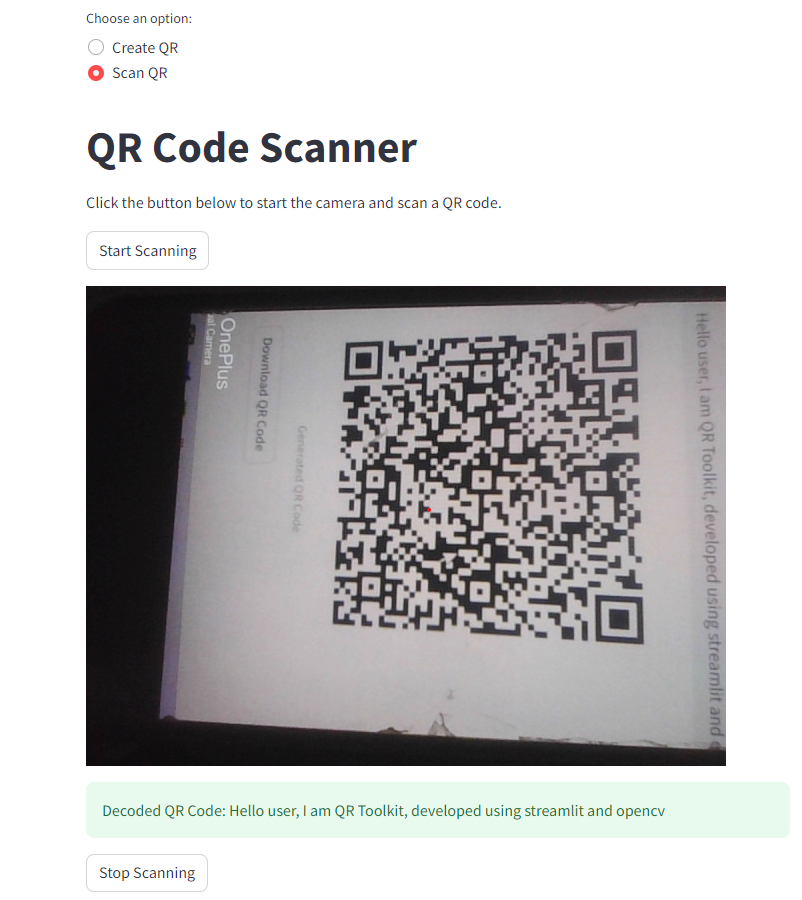
You were reading Streamlit app for QR Toolkit in 6 simple steps
Streamlit is a library offered by python. Similarly, nowadys python is in demands for its collection of data science tools and technologies and streamlit is also called as a data app.
If you wish to learn and curve your career in the data science field feel free to join our free workshop on Masters in Data Science with PowerBI, where you will get to know how exactly the data science field works and why companies are ready to pay handsome salaries in this field.
In this workshop, you will get to know each tool and technology from scratch that will make you skillfully eligible for any data science profile.
To join this workshop, register yourself on consoleflare and we will call you back.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,