Are you preparing for a data science interview? Mastering Python, Pandas, and SQL is critical if you want to stand out. These tools are essential for data analysis, data manipulation, and querying databases, making them a must-have skill set for any data scientist. In this blog, we’ll cover 35 of the most important interview questions on Python, Pandas, and SQL that you need to know. Whether you’re a beginner or brushing up for a high-level role, this guide is for you!
Python Interview Questions for Data Science
1. What is Python? Why is it popular for Data Science?
Python is an interpreted, high-level, general-purpose programming language. It is popular in data science because of its simplicity, ease of use, and vast ecosystem of libraries like Pandas, NumPy, and Scikit-learn, which makes data manipulation, statistical analysis, and machine learning easy.
2. What is a Python list, and how is it different from a tuple?
A list is mutable, meaning you can modify its content (add, remove, update elements), while a tuple is immutable, meaning it cannot be changed once created.
3. What are Python dictionaries?
Dictionaries are ordered collections of data in Python, stored in key-value pairs. They are mutable, meaning their values can be changed.
4. How do you handle exceptions in Python?
Exceptions in Python are handled using the try...except
block. Code that may raise an error is placed inside the try
block, and the error is caught in the except
block.
5. What are lambda functions in Python?
Lambda functions are anonymous, small functions defined using the lambda
keyword. They are typically used for single-line functions and passed as arguments to other functions.
6. How does Python handle memory management?
Python uses an automatic memory management system called a garbage collector, which recycles memory when objects are no longer in use.
7. What is the use of the map()
function in Python?
The map()
function applies a given function to all items in an input list (or iterable) and returns an iterator with the results.
8. What is the __init__
method in Python?__init__
is a constructor method in Python classes. It is used to initialize objects when a new instance of the class is created.
9. How does list comprehension work in Python?
List comprehension provides a concise way to create lists in Python. It replaces the need for loops to append items to a list. Example: [x**2 for x in range(5)]
creates a list of squares from 0 to 4.
10. What is the difference between a while loop and a for loop?
The while
loop keeps executing the block of code as long as the given condition is True
. It is typically used when the number of iterations is not known in advance and depends on a condition.
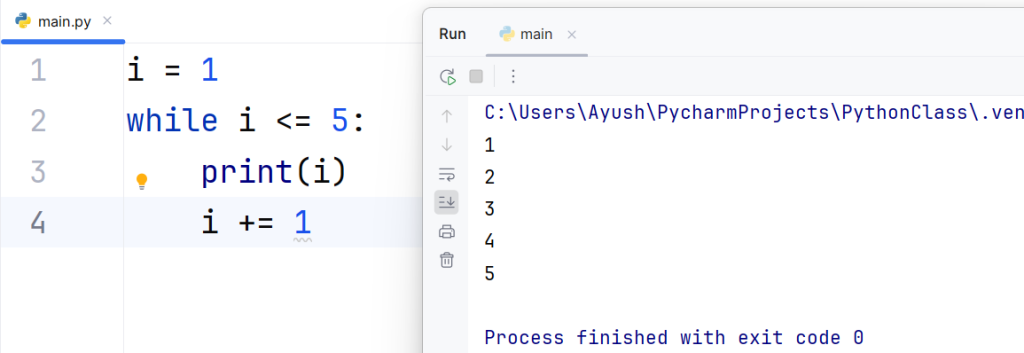
The for
loop iterates over a sequence (such as a list, tuple, string, or range) or any other iterable object. The number of iterations is fixed based on the length of the sequence or range.
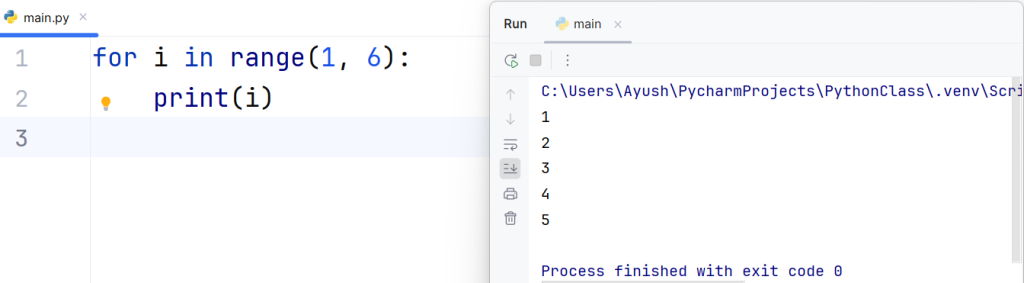
Pandas Interview Questions for Data Science
11. What is Pandas?
Pandas is a powerful open-source data analysis and manipulation library for Python. It provides fast, flexible data structures like DataFrame and Series for managing data efficiently.
12. How do you create a DataFrame in Pandas?
A DataFrame can be created by passing a dictionary, list, or NumPy array to the pd.DataFrame()
function. For example:
import pandas as pd
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]}
df = pd.DataFrame(data)
Python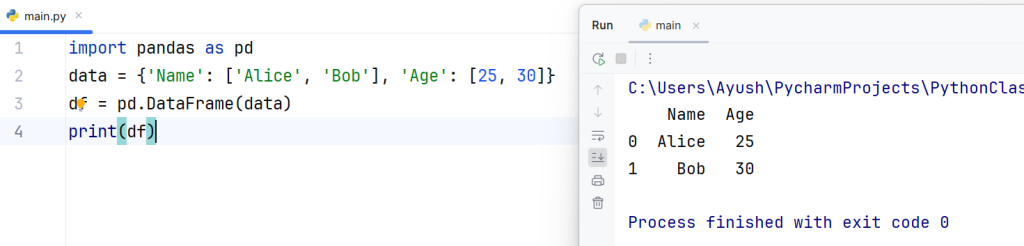
13. How do you handle missing values in a Pandas data frame?
Missing values can be handled using functions like isna()
to detect missing values, fillna()
to replace missing values, and dropna()
to remove rows/columns with missing data.
14. What is the difference between loc
and iloc
in Pandas?
loc
: Accesses rows/columns by labels.iloc
: Accesses rows/columns by index positions.
15. How do you concatenate DataFrames in Pandas?
DataFrames can be concatenated using pd.concat()
for stacking along a particular axis (rows or columns).
For more such content and regular updates, follow us on Facebook, Instagram, LinkedIn
16. How do you group data in Pandas?
Pandas offers the groupby()
function to group data based on one or more columns. You can then apply aggregate functions like sum()
, mean()
, etc.
17. What is a Pandas Series?
A Pandas Series is a one-dimensional labeled array capable of holding any data type. It’s like a column in a data frame.
18. How do you merge DataFrames in Pandas?
DataFrames can be merged using the merge()
function, which behaves similarly to SQL joins (left, right, inner, outer joins).
19. How do you reset the index of a data frame?
Use reset_index()
to reset the index of a data frame and drop=True
to avoid keeping the old index as a column.
20. How do you filter rows in a data frame?
You can filter rows using conditional statements. For example:
df[df['Age'] > 25]
PythonSQL Interview Questions for Data Science
21. What is SQL?
SQL (Structured Query Language) is used for managing and manipulating relational databases by querying, updating, and modifying the data.
22. What are the different types of SQL joins?
- INNER JOIN: Returns records with matching values in both tables.
- LEFT JOIN: Returns all records from the left table and matches records from the right table.
- RIGHT JOIN: Returns all records from the right table and matches records from the left table.
- FULL JOIN: Returns all records when there is a match in either table.
23. What is a primary key?
A primary key is a unique identifier for a record in a table. It ensures that each record is unique and cannot contain null values.
24. What is the difference between DELETE
and TRUNCATE
?
DELETE
: Removes rows one by one and can be rolled back.TRUNCATE
: Deletes all rows in a table instantly and cannot be rolled back.
25. How do you fetch unique records from a table?
You can use the DISTINCT
keyword in the SELECT statement to fetch unique records.
26. What are indexes in SQL, and why are they used?
Indexes are used to speed up database queries by providing a quick lookup of data. However, they may slow down data modification operations like insert and update.
27. How do you join three tables in SQL?
You can join three tables by writing consecutive JOIN
clauses. Example:
SELECT * FROM Table1 JOIN Table2 ON Table1.id = Table2.id JOIN Table3 ON Table2.id = Table3.id;
SQL28. What is normalization in SQL?
Normalization is the process of organizing a database to reduce redundancy and improve data integrity. It involves dividing a database into smaller, related tables.
29. What is a foreign key?
A foreign key is a field in one table that refers to the primary key of another table. It ensures referential integrity between two tables.
30. What is a subquery?
A subquery is a query nested inside another query. It is used to filter data in the outer query.
Why You Should Learn Data Science!
Data Science is one of the most in-demand skills today, spanning a wide array of fields such as e-commerce, healthcare, finance, and more. In this project, you’ve scratched the surface by working with data extraction and automation. Data Science enables businesses to derive insights from raw data, make better decisions, and predict future trends.
By learning data science, you open up career opportunities in fields like machine learning, artificial intelligence, data analysis, and business intelligence. You can build practical tools, analyze large datasets, and create models that drive business success.
If you’re excited about what you can do with Python, data, and automation, then a Data Science course is the perfect next step. Our Data Science course will teach you everything you need to know, from beginner-level Python to advanced data science concepts such as machine learning, statistical modeling, and data visualization.
Read more about DATA SCIENCE
Here’s how a data science course can benefit you:
High Demand: The demand for data scientists continues to grow as organizations increasingly rely on data-driven decision-making.
Diverse Career Paths: Data science skills are applicable in numerous fields, including finance, healthcare, marketing, and technology.
Lucrative Salaries: Data scientists are among the highest-paid professionals in the tech industry.
Continuous Learning: Data science is a field that constantly evolves, offering endless opportunities for growth and learning.
If you’re ready to embark on a rewarding career in data science, consider enrolling in a comprehensive course that focuses on Python.
At ConsoleFlare, we offer tailored courses that provide hands-on experience and in-depth knowledge to help you master Python and excel in your data science journey. Join us and take the first step towards becoming a data science expert with Python at your fingertips.
Register yourself with ConsoleFlare for our free workshop on data science. In this workshop, you will get to know each tool and technology of data analysis from scratch that will make you skillfully eligible for any data science profile.
Thinking, Why Console Flare?
- Recently, ConsoleFlare has been recognized as one of the Top 10 Most Promising Data Science Training Institutes of 2023.
- Console Flare offers the opportunity to learn Data Science in Hindi, just like how you speak daily.
- Console Flare believes in the idea of “What to learn and what not to learn” and this can be seen in their curriculum structure. They have designed their program based on what you need to learn for data science and nothing else.
- Want more reasons,